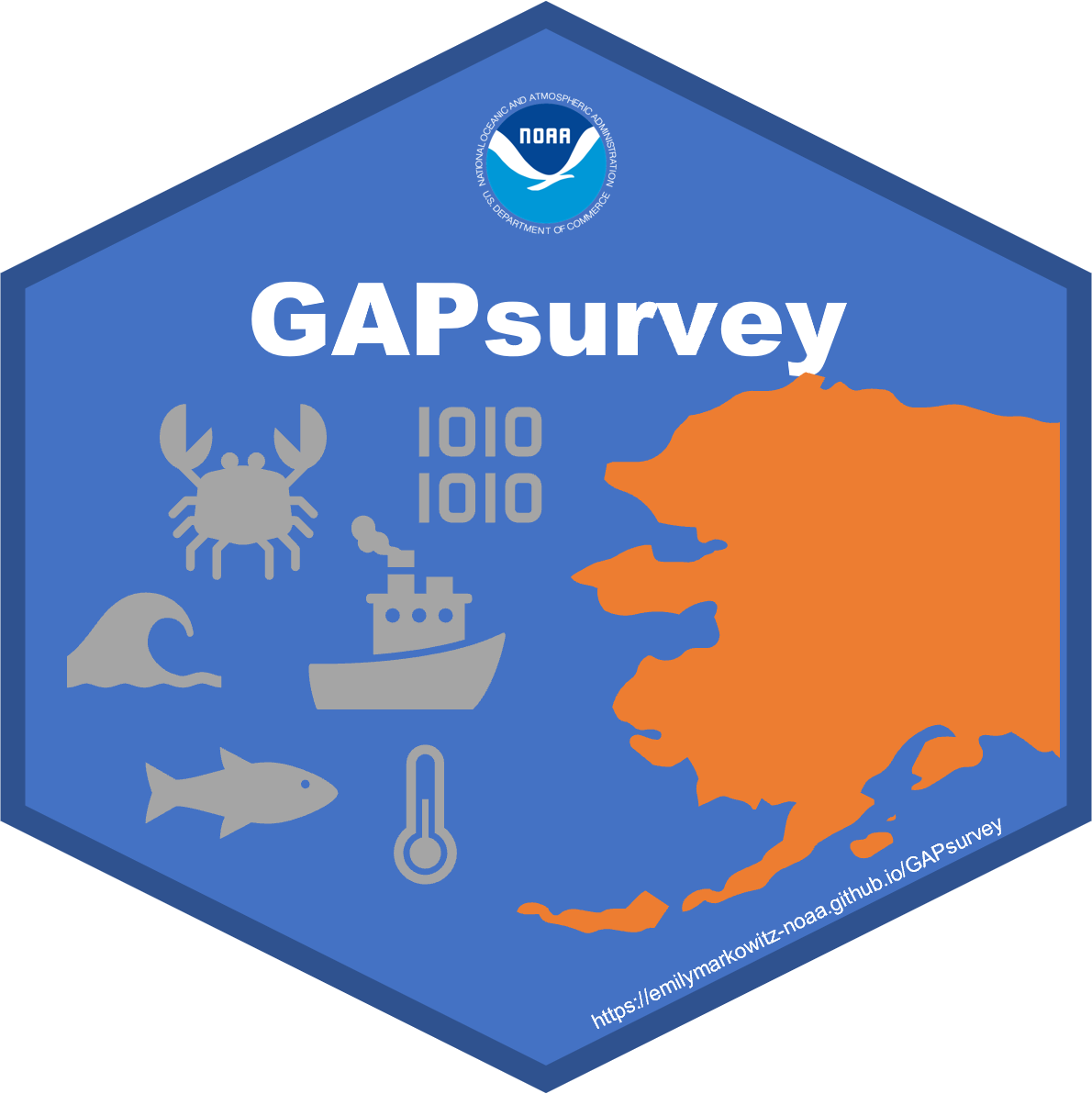
Find historical catch data from previous years
get_catch_haul_history.Rd
Find historical catch data from previous years
Arguments
- survey
(character) A character string of the survey you are interested in reivewing. Options are those from public_data$survey, which are "AI", "GOA", "EBS", "NBS", "BSS".
- species_codes
(numeric) A species code number of a species or species you are specifically interested in reviewing data from. If NA/not entered, the function will return data for all species caught in the haul.
- years
(numeric) the years you want returned in the output. If years = NA, script will default to the last 10 years. If you would like to see all years, simply choose a large range that covers all years of the survey (e.g., 1970:2030)
- station
(character) A character string of the current station name (as a grid cell; e.g., "264-85")
- grid_buffer
(numeric) GOA/AI only. The number of cells around the current station where you would like to see catches from. Typically, use grid_buffer = 3.
Examples
#' # EBS (or NBS) --------------------------------------------------------------
## for one year and only 1 station for all species --------------------------
get_catch_haul_history(
survey = "EBS",
years = 2021,
station = "I-13")
#> $catch
#> $catch$`2021`
#> station scientific_name common_name count
#> 1 I-13 Gadus chalcogrammus walleye pollock 793
#> 2 I-13 Lepidopsetta polyxystra northern rock sole 1530
#> 3 I-13 Limanda aspera yellowfin sole 584
#> 4 I-13 Asterias amurensis purple-orange sea star 1816
#> 5 I-13 Arctoraja parmifera Alaska skate 6
#> 6 I-13 Gadus macrocephalus Pacific cod 91
#> 7 I-13 Hippoglossus stenolepis Pacific halibut 45
#> 8 I-13 Hippoglossoides elassodon flathead sole 48
#> 9 I-13 Paralithodes camtschaticus red king crab 18
#> 10 I-13 Pleuronectes quadrituberculatus Alaska plaice 47
#> 11 I-13 Myoxocephalus jaok plain sculpin 3
#> 12 I-13 Neptunea sp. <NA> 35
#> 13 I-13 Isopsetta isolepis butter sole 17
#> 14 I-13 Podothecus accipenserinus sturgeon poacher 54
#> 15 I-13 Platichthys stellatus starry flounder 2
#> 16 I-13 Porifera sponge unid. NA
#> 17 I-13 Gorgonocephalus eucnemis basketstar 8
#> 18 I-13 Pagurus ochotensis Alaskan hermit 8
#> 19 I-13 <NA> empty gastropod shells NA
#> 20 I-13 Mactromeris sp. <NA> 8
#> 21 I-13 Ascidiacea tunicate unid. NA
#> 22 I-13 Hyas lyratus Pacific lyre crab 6
#> 23 I-13 <NA> empty bivalve shells NA
#> 24 I-13 Clupea pallasii Pacific herring 2
#> 25 I-13 Chionoecetes bairdi Tanner crab 1
#> 26 I-13 Actiniaria sea anemone unid. 8
#> 27 I-13 Scyphozoa jellyfish unid. 2
#> 28 I-13 Octocorallia soft coral unid. NA
#> 29 I-13 Liparis sp. <NA> 2
#> 30 I-13 Polychaeta polychaete worm unid. 4
#> 31 I-13 Glebocarcinus oregonensis Oregon rock crab 2
#> 32 I-13 Crangon sp. <NA> 2
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 1 550.359 11529.782133 16613.00575
#> 2 213.721 4477.362171 32052.83581
#> 3 139.534 2923.176727 12234.54648
#> 4 127.104 2662.773623 38044.41166
#> 5 41.960 879.043785 125.69740
#> 6 31.140 652.369482 1906.41050
#> 7 26.103 546.846518 942.73046
#> 8 23.811 498.830113 1005.57916
#> 9 19.380 406.002587 377.09219
#> 10 16.947 355.032293 984.62960
#> 11 5.420 113.546647 62.84870
#> 12 4.401 92.199039 733.23481
#> 13 4.072 85.306632 356.14262
#> 14 3.355 70.285794 1131.27656
#> 15 3.219 67.436653 41.89913
#> 16 2.889 60.523296 0.00000
#> 17 1.861 38.987142 167.59653
#> 18 0.853 17.869980 167.59653
#> 19 0.795 16.654905 0.00000
#> 20 0.620 12.988731 167.59653
#> 21 0.504 10.558581 0.00000
#> 22 0.465 9.741548 125.69740
#> 23 0.427 8.945465 0.00000
#> 24 0.339 7.101903 41.89913
#> 25 0.220 4.608904 20.94957
#> 26 0.213 4.462258 167.59653
#> 27 0.175 3.666174 41.89913
#> 28 0.068 1.424570 0.00000
#> 29 0.019 0.398042 41.89913
#> 30 0.010 0.209496 83.79826
#> 31 0.010 0.209496 41.89913
#> 32 0.004 0.083798 41.89913
#>
#>
#> $catch_means
#> [1] "A summary of catch data would not be helpful with these function parameters"
#>
#> $haul
#> year station haul stratum vessel_name date_time latitude_dd_start
#> 1 2021 I-13 7 31 ALASKA KNIGHT 2021-06-02 12:05:29 57.65493
#> longitude_dd_start bottom_temperature_c surface_temperature_c depth_m
#> 1 -160.2535 4.4 4.7 55
#> distance_fished_km net_width_m net_height_m area_swept_km2 duration_hr
#> 1 2.954 16.159 1.794 0.04773369 0.522
#> total_weight_kg
#> 1 1220
#>
## for default 10 years and only 1 station for PCOD and walleye pollock ----
get_catch_haul_history(
species_codes = c(21720, 21740), # pacific cod and walleye pollock
survey = "EBS",
station = "I-13")
#> $catch
#> $catch$`2014`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 19 I-13 Gadus macrocephalus Pacific cod 138 54.367 1306.6566
#> 20 I-13 Gadus chalcogrammus walleye pollock 65 41.508 997.6033
#> cpue_nokm2
#> 19 3316.692
#> 20 1562.210
#>
#> $catch$`2015`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 17 I-13 Gadus macrocephalus Pacific cod 827 442.790 9202.058
#> 18 I-13 Gadus chalcogrammus walleye pollock 363 377.003 7834.873
#> cpue_nokm2
#> 17 17186.707
#> 18 7543.863
#>
#> $catch$`2016`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 15 I-13 Gadus macrocephalus Pacific cod 779 776.725 16690.3179
#> 16 I-13 Gadus chalcogrammus walleye pollock 37 7.043 151.3404
#> cpue_nokm2
#> 15 16739.2032
#> 16 795.0584
#>
#> $catch$`2017`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 13 I-13 Gadus chalcogrammus walleye pollock 139 57.062 1303.8383
#> 14 I-13 Gadus macrocephalus Pacific cod 13 9.800 223.9251
#> cpue_nokm2
#> 13 3176.0808
#> 14 297.0435
#>
#> $catch$`2018`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 11 I-13 Gadus chalcogrammus walleye pollock 95 80.05 1685.4747
#> 12 I-13 Gadus macrocephalus Pacific cod 8 5.05 106.3291
#> cpue_nokm2
#> 11 2000.2511
#> 12 168.4422
#>
#> $catch$`2019`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 9 I-13 Gadus macrocephalus Pacific cod 118 43.080 946.3481
#> 10 I-13 Gadus chalcogrammus walleye pollock 31 6.915 151.9034
#> cpue_nokm2
#> 9 2592.133
#> 10 680.984
#>
#> $catch$`2021`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 7 I-13 Gadus chalcogrammus walleye pollock 793 550.359 11529.7821
#> 8 I-13 Gadus macrocephalus Pacific cod 91 31.140 652.3695
#> cpue_nokm2
#> 7 16613.01
#> 8 1906.41
#>
#> $catch$`2022`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 5 I-13 Gadus chalcogrammus walleye pollock 135 140.138 3252.886
#> 6 I-13 Gadus macrocephalus Pacific cod 173 104.016 2414.421
#> cpue_nokm2
#> 5 3133.623
#> 6 4015.679
#>
#> $catch$`2023`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 3 I-13 Gadus chalcogrammus walleye pollock 70 41.744 898.023
#> 4 I-13 Gadus macrocephalus Pacific cod 31 9.190 197.701
#> cpue_nokm2
#> 3 1505.8837
#> 4 666.8913
#>
#> $catch$`2024`
#> station scientific_name common_name count weight_kg cpue_kgkm2
#> 1 I-13 Gadus chalcogrammus walleye pollock 353 351.285 8045.493
#> 2 I-13 Gadus macrocephalus Pacific cod 118 48.125 1102.209
#> cpue_nokm2
#> 1 8084.771
#> 2 2702.558
#>
#>
#> $catch_means
#> scientific_name common_name station count weight_kg cpue_kgkm2
#> 1 Gadus chalcogrammus walleye pollock I-13 208.1 165.31 3585.12
#> 2 Gadus macrocephalus Pacific cod I-13 229.6 152.43 3284.23
#> cpue_nokm2 Freq
#> 1 4509.57 10
#> 2 4959.18 10
#>
#> $haul
#> year station haul stratum vessel_name date_time
#> 1 2014 I-13 8 31 ALASKA KNIGHT 2014-06-09 12:34:43
#> 2 2015 I-13 9 31 VESTERAALEN 2015-06-04 11:36:07
#> 3 2016 I-13 12 31 ALASKA KNIGHT 2016-06-02 12:23:54
#> 4 2017 I-13 8 31 ALASKA KNIGHT 2017-06-05 15:12:37
#> 5 2018 I-13 9 31 ALASKA KNIGHT 2018-06-04 12:39:27
#> 6 2019 I-13 11 31 VESTERAALEN 2019-06-04 13:15:57
#> 7 2021 I-13 7 31 ALASKA KNIGHT 2021-06-02 12:05:29
#> 8 2022 I-13 12 31 VESTERAALEN 2022-06-01 16:20:20
#> 9 2023 I-13 16 31 NORTHWEST EXPLORER 2023-05-30 13:43:02
#> 10 2024 I-13 31 31 NORTHWEST EXPLORER 2024-06-05 15:40:19
#> latitude_dd_start longitude_dd_start bottom_temperature_c
#> 1 57.67458 -160.2594 5.0
#> 2 57.67938 -160.2449 4.6
#> 3 57.66506 -160.2658 6.0
#> 4 57.65211 -160.2444 3.3
#> 5 57.68093 -160.2568 4.3
#> 6 57.69052 -160.2580 5.5
#> 7 57.65493 -160.2535 4.4
#> 8 57.65502 -160.2754 3.6
#> 9 57.66505 -160.2725 3.4
#> 10 57.64550 -160.2743 3.0
#> surface_temperature_c depth_m distance_fished_km net_width_m net_height_m
#> 1 6.1 54 2.772 15.010 2.257
#> 2 7.5 55 2.969 16.207 2.635
#> 3 7.1 55 3.116 14.935 2.880
#> 4 3.5 53 2.829 15.470 2.426
#> 5 4.5 54 2.887 16.451 2.459
#> 6 7.5 54 2.840 16.029 2.200
#> 7 4.7 55 2.954 16.159 1.794
#> 8 7.6 54 2.854 15.095 2.566
#> 9 3.4 55 2.879 16.146 2.175
#> 10 3.3 53 2.796 15.616 2.287
#> area_swept_km2 duration_hr total_weight_kg
#> 1 0.04160772 0.503 1456.00
#> 2 0.04811858 0.527 3026.00
#> 3 0.04653746 0.545 4480.00
#> 4 0.04376463 0.518 1296.00
#> 5 0.04749404 0.515 875.12
#> 6 0.04552236 0.520 3186.00
#> 7 0.04773369 0.522 1220.00
#> 8 0.04308113 0.531 1190.00
#> 9 0.04648433 0.516 812.00
#> 10 0.04366234 0.511 2893.00
#>
# AI (or GOA) ---------------------------------------------------------------
## for two specific years and nearby stations -------------------------------
get_catch_haul_history(
survey = "AI",
years = c(2016, 2018),
station = "324-73",
grid_buffer = 3)
#> $catch
#> $catch$`2016`
#> haul scientific_name common_name station
#> 82 3 Gadus macrocephalus Pacific cod 320-73
#> 83 7 Atheresthes stomias arrowtooth flounder 320-74
#> 84 3 Hippoglossus stenolepis Pacific halibut 320-73
#> 85 5 Hippoglossoides elassodon flathead sole 320-74
#> 86 5 Lepidopsetta bilineata southern rock sole 320-74
#> 87 3 Lepidopsetta bilineata southern rock sole 320-73
#> 88 3 Lepidopsetta polyxystra northern rock sole 320-73
#> 89 5 Hippoglossus stenolepis Pacific halibut 320-74
#> 90 4 Hippoglossoides elassodon flathead sole 324-73
#> 91 7 Gadus macrocephalus Pacific cod 320-74
#> 92 4 Atheresthes stomias arrowtooth flounder 324-73
#> 93 7 Lepidopsetta polyxystra northern rock sole 320-74
#> 94 3 Atheresthes stomias arrowtooth flounder 320-73
#> 95 7 Hippoglossus stenolepis Pacific halibut 320-74
#> 96 4 Hippoglossus stenolepis Pacific halibut 324-73
#> 97 7 Enteroctopus dofleini giant octopus 320-74
#> 98 5 Lepidopsetta polyxystra northern rock sole 320-74
#> 99 4 Anoplopoma fimbria sablefish 324-73
#> 100 4 Gadus chalcogrammus walleye pollock 324-73
#> 101 4 Lepidopsetta bilineata southern rock sole 324-73
#> 102 5 Atheresthes stomias arrowtooth flounder 320-74
#> 103 4 Parophrys vetulus English sole 324-73
#> 104 4 Glyptocephalus zachirus rex sole 324-73
#> 105 5 Gadus macrocephalus Pacific cod 320-74
#> 106 3 Anarrhichthys ocellatus wolf-eel 320-73
#> 107 4 Enteroctopus dofleini giant octopus 324-73
#> 108 4 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 109 3 Clupea pallasii Pacific herring 320-73
#> 110 7 Hemilepidotus jordani yellow Irish lord 320-74
#> 111 3 Pleurogrammus monopterygius Atka mackerel 320-73
#> 112 7 Glyptocephalus zachirus rex sole 320-74
#> 113 5 Hemilepidotus jordani yellow Irish lord 320-74
#> 114 7 Lepidopsetta bilineata southern rock sole 320-74
#> 115 4 Gadus macrocephalus Pacific cod 324-73
#> 116 7 Atheresthes evermanni Kamchatka flounder 320-74
#> 117 3 Hemilepidotus jordani yellow Irish lord 320-73
#> 118 5 Pycnopodia helianthoides sunflower sea star 320-74
#> 119 4 Gorgonocephalus eucnemis basketstar 324-73
#> 120 4 Thaliacea salp unid. 324-73
#> 121 3 Hippoglossoides elassodon flathead sole 320-73
#> 122 5 Pleurogrammus monopterygius Atka mackerel 320-74
#> 123 7 Sebastes polyspinis northern rockfish 320-74
#> 124 5 Gadus chalcogrammus walleye pollock 320-74
#> 125 7 Hippoglossoides elassodon flathead sole 320-74
#> 126 4 Atheresthes evermanni Kamchatka flounder 324-73
#> 127 4 Podothecus accipenserinus sturgeon poacher 324-73
#> 128 3 Pennatuloidea sea whip or sea pen unid. 320-73
#> 129 5 Gorgonocephalus eucnemis basketstar 320-74
#> 130 4 Sebastes polyspinis northern rockfish 324-73
#> 131 7 Pagurus trigonocheirus fuzzy hermit crab 320-74
#> 132 7 Thaliacea salp unid. 320-74
#> 133 4 Bathymaster signatus searcher 324-73
#> 134 7 Elassochirus cavimanus purple hermit 320-74
#> 135 4 Pagurus confragosus knobbyhand hermit 324-73
#> 136 7 Modiolus modiolus northern horsemussel 320-74
#> 137 5 Thaliacea salp unid. 320-74
#> 138 3 Ammodytes sp. sand lance unid. 320-73
#> 139 4 Pagurus aleuticus Aleutian hermit 324-73
#> 140 7 Pagurus confragosus knobbyhand hermit 320-74
#> 141 4 Porifera sponge unid. 324-73
#> 142 7 Ceramaster patagonicus orange bat sea star 320-74
#> 143 3 Gorgonocephalus eucnemis basketstar 320-73
#> 144 4 Polychaeta polychaete worm unid. 324-73
#> 145 5 Rajiformes egg case skate egg case unid. 320-74
#> 146 7 Bryozoa bryozoan unid. 320-74
#> 147 4 Nudibranchia nudibranch unid. 324-73
#> 148 5 Ophiuroidea brittlestar unid. 320-74
#> 149 3 Ophiuroidea brittlestar unid. 320-73
#> 150 7 Actiniaria sea anemone unid. 320-74
#> 151 4 Rajiformes egg case skate egg case unid. 324-73
#> 152 7 Hyas lyratus Pacific lyre crab 320-74
#> 153 4 Actiniaria sea anemone unid. 324-73
#> 154 4 Labidochirus splendescens splendid hermit 324-73
#> 155 7 Rajiformes egg case skate egg case unid. 320-74
#> 156 7 Ascidiacea tunicate unid. 320-74
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 82 243 343.340 14793.441181 10470.10604
#> 83 291 136.844 6214.554539 13215.30627
#> 84 133 118.374 5100.363507 5730.55186
#> 85 78 67.768 3050.003623 3510.51060
#> 86 69 62.926 2832.081926 3105.45169
#> 87 90 59.940 2582.626156 3877.81705
#> 88 103 33.600 1447.718366 4437.94618
#> 89 31 28.166 1267.654380 1395.20293
#> 90 133 23.320 950.181635 5419.13196
#> 91 10 20.740 941.874405 454.13424
#> 92 54 20.212 823.545077 2200.24907
#> 93 41 20.080 911.901546 1861.95037
#> 94 27 17.950 773.409067 1163.34512
#> 95 12 15.040 683.017891 544.96108
#> 96 11 10.780 439.234907 448.19888
#> 97 2 10.220 464.125189 90.82685
#> 98 26 9.904 445.744834 1170.17020
#> 99 11 8.980 365.893271 448.19888
#> 100 10 8.680 353.669665 407.45353
#> 101 6 8.620 351.224944 244.47212
#> 102 8 8.320 374.454464 360.05237
#> 103 6 7.000 285.217472 244.47212
#> 104 29 6.612 269.408275 1181.61524
#> 105 4 6.080 273.639801 180.02618
#> 106 1 5.900 254.212451 43.08686
#> 107 1 5.562 226.625654 40.74535
#> 108 1 4.960 202.096951 40.74535
#> 109 10 4.320 186.135218 430.86856
#> 110 6 3.920 178.020621 272.48054
#> 111 5 3.870 166.746133 215.43428
#> 112 7 3.340 151.680835 317.89396
#> 113 2 2.540 114.316627 90.01309
#> 114 2 2.468 112.080329 90.82685
#> 115 2 2.240 91.269591 81.49071
#> 116 8 1.672 75.931244 363.30739
#> 117 1 1.332 57.391692 43.08686
#> 118 1 1.320 59.408641 45.00655
#> 119 4 1.182 48.161007 162.98141
#> 120 NA 1.072 43.679019 0.00000
#> 121 1 0.946 40.760166 43.08686
#> 122 1 0.910 40.955957 45.00655
#> 123 2 0.863 39.191785 90.82685
#> 124 1 0.754 33.934936 45.00655
#> 125 1 0.648 29.427898 45.41342
#> 126 5 0.416 16.950067 203.72677
#> 127 4 0.360 14.668327 162.98141
#> 128 NA 0.258 11.116409 0.00000
#> 129 1 0.236 10.621545 45.00655
#> 130 1 0.198 8.067580 40.74535
#> 131 5 0.186 8.446897 227.06712
#> 132 NA 0.172 7.811109 0.00000
#> 133 1 0.152 6.193294 40.74535
#> 134 5 0.148 6.721187 227.06712
#> 135 5 0.142 5.785840 203.72677
#> 136 1 0.090 4.087208 45.41342
#> 137 NA 0.088 3.960576 0.00000
#> 138 2 0.080 3.446948 86.17371
#> 139 3 0.066 2.689193 122.23606
#> 140 2 0.060 2.724805 90.82685
#> 141 NA 0.060 2.444721 0.00000
#> 142 1 0.058 2.633979 45.41342
#> 143 1 0.056 2.412864 43.08686
#> 144 NA 0.054 2.200249 0.00000
#> 145 4 0.048 2.160314 180.02618
#> 146 NA 0.044 1.998191 0.00000
#> 147 1 0.036 1.466833 40.74535
#> 148 6 0.032 1.440209 270.03928
#> 149 5 0.020 0.861737 215.43428
#> 150 1 0.020 0.908268 45.41342
#> 151 NA 0.020 0.814907 0.00000
#> 152 2 0.018 0.817442 90.82685
#> 153 1 0.016 0.651926 40.74535
#> 154 1 0.008 0.325963 40.74535
#> 155 NA 0.004 0.181654 0.00000
#> 156 NA 0.002 0.090827 0.00000
#>
#> $catch$`2018`
#> haul scientific_name common_name station count
#> 1 2 Gadus chalcogrammus walleye pollock 325-76 1616
#> 2 9 Atheresthes stomias arrowtooth flounder 320-74 1041
#> 3 1 Gadus chalcogrammus walleye pollock 324-73 489
#> 4 2 Porifera sponge unid. 325-76 NA
#> 5 1 Atheresthes stomias arrowtooth flounder 324-73 357
#> 6 9 Lepidopsetta polyxystra northern rock sole 320-74 99
#> 7 2 Sebastes polyspinis northern rockfish 325-76 88
#> 8 2 Gadus macrocephalus Pacific cod 325-76 12
#> 9 9 Gadus chalcogrammus walleye pollock 320-74 35
#> 10 9 Gadus macrocephalus Pacific cod 320-74 9
#> 11 2 Atheresthes stomias arrowtooth flounder 325-76 33
#> 12 9 Hemilepidotus jordani yellow Irish lord 320-74 29
#> 13 9 Hippoglossus stenolepis Pacific halibut 320-74 9
#> 14 9 Bathyraja aleutica Aleutian skate 320-74 1
#> 15 1 Hippoglossus stenolepis Pacific halibut 324-73 11
#> 16 9 Sebastes alutus Pacific ocean perch 320-74 40
#> 17 1 Gadus macrocephalus Pacific cod 324-73 6
#> 18 2 Lepidopsetta polyxystra northern rock sole 325-76 54
#> 19 10 Gadus macrocephalus Pacific cod 320-73 4
#> 20 2 Enteroctopus dofleini giant octopus 325-76 1
#> 21 10 Hippoglossus stenolepis Pacific halibut 320-73 6
#> 22 2 Hemilepidotus jordani yellow Irish lord 325-76 17
#> 23 2 Hexagrammos decagrammus kelp greenling 325-76 30
#> 24 1 Hippoglossoides elassodon flathead sole 324-73 37
#> 25 2 Hippoglossus stenolepis Pacific halibut 325-76 5
#> 26 9 Sebastes polyspinis northern rockfish 320-74 14
#> 27 9 Glyptocephalus zachirus rex sole 320-74 13
#> 28 9 Lepidopsetta bilineata southern rock sole 320-74 9
#> 29 1 Chionoecetes bairdi Tanner crab 324-73 25
#> 30 1 Glyptocephalus zachirus rex sole 324-73 24
#> 31 2 Pleurogrammus monopterygius Atka mackerel 325-76 10
#> 32 9 Enteroctopus dofleini giant octopus 320-74 1
#> 33 9 Hippoglossoides elassodon flathead sole 320-74 8
#> 34 10 Anarrhichthys ocellatus wolf-eel 320-73 1
#> 35 9 Pleurogrammus monopterygius Atka mackerel 320-74 3
#> 36 10 Lepidopsetta bilineata southern rock sole 320-73 7
#> 37 1 Arctoraja parmifera Alaska skate 324-73 1
#> 38 9 Atheresthes evermanni Kamchatka flounder 320-74 3
#> 39 2 Hippoglossoides elassodon flathead sole 325-76 4
#> 40 2 Myoxocephalus polyacanthocephalus great sculpin 325-76 1
#> 41 1 Pycnopodia helianthoides sunflower sea star 324-73 1
#> 42 2 Lepidopsetta bilineata southern rock sole 325-76 8
#> 43 2 Sebastes alutus Pacific ocean perch 325-76 30
#> 44 1 Myoxocephalus polyacanthocephalus great sculpin 324-73 1
#> 45 1 Bathyraja interrupta Bering skate 324-73 1
#> 46 2 Sebastes variabilis dusky rockfish 325-76 4
#> 47 2 Zaprora silenus prowfish 325-76 1
#> 48 10 Pleurogrammus monopterygius Atka mackerel 320-73 1
#> 49 2 Holothuroidea sea cucumber unid. 325-76 3
#> 50 1 Sebastes polyspinis northern rockfish 324-73 2
#> 51 1 Anoplopoma fimbria sablefish 324-73 2
#> 52 1 Hemilepidotus jordani yellow Irish lord 324-73 1
#> 53 2 Bathymaster signatus searcher 325-76 2
#> 54 10 Atheresthes stomias arrowtooth flounder 320-73 2
#> 55 1 Scyphozoa jellyfish unid. 324-73 4
#> 56 1 Thaliacea salp unid. 324-73 5
#> 57 1 Pagurus ochotensis Alaskan hermit 324-73 15
#> 58 1 Gorgonocephalus eucnemis basketstar 324-73 1
#> 59 1 Actiniaria sea anemone unid. 324-73 4
#> 60 2 Modiolus modiolus northern horsemussel 325-76 12
#> 61 9 Scyphozoa jellyfish unid. 320-74 1
#> 62 1 Triglops macellus roughspine sculpin 324-73 5
#> 63 2 Crystallichthys cyclospilus blotched snailfish 325-76 1
#> 64 2 Ceramaster patagonicus orange bat sea star 325-76 4
#> 65 1 Lepidopsetta polyxystra northern rock sole 324-73 1
#> 66 2 Gorgonocephalus eucnemis basketstar 325-76 2
#> 67 1 Sarritor frenatus sawback poacher 324-73 3
#> 68 2 Scyphozoa jellyfish unid. 325-76 2
#> 69 2 Elassochirus gilli Pacific red hermit 325-76 2
#> 70 2 Podothecus accipenserinus sturgeon poacher 325-76 1
#> 71 2 Nudibranchia nudibranch unid. 325-76 2
#> 72 1 Atheresthes evermanni Kamchatka flounder 324-73 1
#> 73 1 Oregonia gracilis graceful decorator crab 324-73 1
#> 74 1 Porifera sponge unid. 324-73 NA
#> 75 2 Pagurus brandti sponge hermit 325-76 2
#> 76 2 Sarritor frenatus sawback poacher 325-76 1
#> 77 1 Crossaster papposus rose sea star 324-73 1
#> 78 1 Pagurus capillatus hairy hermit crab 324-73 1
#> 79 2 Ophiuroidea brittlestar unid. 325-76 2
#> 80 2 Elassochirus tenuimanus widehand hermit crab 325-76 1
#> 81 1 Ciliatocardium ciliatum hairy cockle 324-73 1
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 1 1211.290 56195.947836 74971.84960
#> 2 643.495 25096.701051 40599.64070
#> 3 399.642 15729.789050 19246.89308
#> 4 322.619 14967.415313 0.00000
#> 5 98.418 3873.702911 14051.41274
#> 6 50.810 1981.621272 3861.06093
#> 7 45.950 2131.780006 4082.62547
#> 8 42.680 1980.073355 556.72166
#> 9 28.595 1115.222599 1365.02154
#> 10 26.500 1033.516310 351.00554
#> 11 23.050 1069.369513 1530.98455
#> 12 20.740 808.872765 1131.01785
#> 13 18.849 735.122601 351.00554
#> 14 18.780 732.431559 39.00062
#> 15 18.470 726.973651 432.95670
#> 16 17.560 684.850808 1560.02462
#> 17 15.540 611.649731 236.15820
#> 18 13.025 604.274964 2505.24745
#> 19 11.950 534.815361 179.01769
#> 20 11.130 516.359335 46.39347
#> 21 9.534 426.688674 268.52654
#> 22 9.350 433.778957 788.68901
#> 23 9.346 433.593383 1391.80414
#> 24 9.250 364.077221 1456.30888
#> 25 9.080 421.252719 231.96736
#> 26 8.980 350.225527 546.00862
#> 27 7.520 293.284628 507.00800
#> 28 7.370 287.434536 351.00554
#> 29 6.900 271.581927 983.99249
#> 30 6.170 242.849346 944.63279
#> 31 5.740 266.298525 463.93471
#> 32 5.380 209.823311 39.00062
#> 33 4.875 190.128000 312.00492
#> 34 4.670 209.003158 44.75442
#> 35 4.300 167.702647 117.00185
#> 36 4.100 183.493136 313.28096
#> 37 3.580 140.907724 39.35970
#> 38 2.940 114.661809 117.00185
#> 39 2.914 135.190575 185.57388
#> 40 2.720 126.190242 46.39347
#> 41 2.504 98.556688 39.35970
#> 42 2.259 104.802852 371.14777
#> 43 2.159 100.163505 1391.80414
#> 44 1.890 74.389832 39.35970
#> 45 1.850 72.815444 39.35970
#> 46 1.632 75.714145 185.57388
#> 47 1.592 73.858406 46.39347
#> 48 1.140 51.020043 44.75442
#> 49 0.994 46.115110 139.18041
#> 50 0.990 38.966103 78.71940
#> 51 0.974 38.336347 78.71940
#> 52 0.880 34.636536 39.35970
#> 53 0.756 35.073464 92.78694
#> 54 0.730 32.670729 89.50885
#> 55 0.576 22.671187 157.43880
#> 56 0.452 17.790584 196.79850
#> 57 0.442 17.396987 590.39549
#> 58 0.430 16.924671 39.35970
#> 59 0.394 15.507722 157.43880
#> 60 0.375 17.397552 556.72166
#> 61 0.295 11.505182 39.00062
#> 62 0.292 11.493032 196.79850
#> 63 0.222 10.299351 46.39347
#> 64 0.200 9.278694 185.57388
#> 65 0.174 6.848588 39.35970
#> 66 0.145 6.727053 92.78694
#> 67 0.140 5.510358 118.07910
#> 68 0.095 4.407380 92.78694
#> 69 0.085 3.943445 92.78694
#> 70 0.072 3.340330 46.39347
#> 71 0.055 2.551641 92.78694
#> 72 0.042 1.653107 39.35970
#> 73 0.042 1.653107 39.35970
#> 74 0.033 1.298870 0.00000
#> 75 0.030 1.391804 92.78694
#> 76 0.024 1.113443 46.39347
#> 77 0.014 0.551036 39.35970
#> 78 0.012 0.472316 39.35970
#> 79 0.005 0.231967 92.78694
#> 80 0.005 0.231967 46.39347
#> 81 0.002 0.078719 39.35970
#>
#>
#> $catch_means
#> scientific_name common_name station count
#> 1 Gadus chalcogrammus walleye pollock 325-76 1616.0
#> 2 Porifera sponge unid. 325-76 NaN
#> 3 Atheresthes stomias arrowtooth flounder 320-74 446.7
#> 4 Gadus chalcogrammus walleye pollock 324-73 249.5
#> 5 Gadus macrocephalus Pacific cod 320-73 123.5
#> 6 Hippoglossus stenolepis Pacific halibut 320-73 69.5
#> 7 Atheresthes stomias arrowtooth flounder 324-73 205.5
#> 8 Sebastes polyspinis northern rockfish 325-76 88.0
#> 9 Gadus macrocephalus Pacific cod 325-76 12.0
#> 10 Lepidopsetta polyxystra northern rock sole 320-73 103.0
#> 11 Lepidopsetta bilineata southern rock sole 320-73 48.5
#> 12 Lepidopsetta polyxystra northern rock sole 320-74 55.3
#> 13 Hippoglossoides elassodon flathead sole 320-74 29.0
#> 14 Lepidopsetta bilineata southern rock sole 320-74 26.7
#> 15 Atheresthes stomias arrowtooth flounder 325-76 33.0
#> 16 Hippoglossus stenolepis Pacific halibut 320-74 17.3
#> 17 Gadus macrocephalus Pacific cod 320-74 7.7
#> 18 Bathyraja aleutica Aleutian skate 320-74 1.0
#> 19 Sebastes alutus Pacific ocean perch 320-74 40.0
#> 20 Hippoglossoides elassodon flathead sole 324-73 85.0
#> 21 Lepidopsetta polyxystra northern rock sole 325-76 54.0
#> 22 Hippoglossus stenolepis Pacific halibut 324-73 11.0
#> 23 Gadus chalcogrammus walleye pollock 320-74 18.0
#> 24 Enteroctopus dofleini giant octopus 325-76 1.0
#> 25 Hemilepidotus jordani yellow Irish lord 325-76 17.0
#> 26 Hexagrammos decagrammus kelp greenling 325-76 30.0
#> 27 Hippoglossus stenolepis Pacific halibut 325-76 5.0
#> 28 Atheresthes stomias arrowtooth flounder 320-73 14.5
#> 29 Hemilepidotus jordani yellow Irish lord 320-74 12.3
#> 30 Gadus macrocephalus Pacific cod 324-73 4.0
#> 31 Lepidopsetta bilineata southern rock sole 324-73 6.0
#> 32 Enteroctopus dofleini giant octopus 320-74 1.5
#> 33 Parophrys vetulus English sole 324-73 6.0
#> 34 Chionoecetes bairdi Tanner crab 324-73 25.0
#> 35 Pleurogrammus monopterygius Atka mackerel 325-76 10.0
#> 36 Glyptocephalus zachirus rex sole 324-73 26.5
#> 37 Anarrhichthys ocellatus wolf-eel 320-73 1.0
#> 38 Enteroctopus dofleini giant octopus 324-73 1.0
#> 39 Glyptocephalus zachirus rex sole 320-74 10.0
#> 40 Anoplopoma fimbria sablefish 324-73 6.5
#> 41 Sebastes polyspinis northern rockfish 320-74 8.0
#> 42 Clupea pallasii Pacific herring 320-73 10.0
#> 43 Arctoraja parmifera Alaska skate 324-73 1.0
#> 44 Myoxocephalus polyacanthocephalus great sculpin 324-73 1.0
#> 45 Hippoglossoides elassodon flathead sole 325-76 4.0
#> 46 Myoxocephalus polyacanthocephalus great sculpin 325-76 1.0
#> 47 Pleurogrammus monopterygius Atka mackerel 320-73 3.0
#> 48 Lepidopsetta bilineata southern rock sole 325-76 8.0
#> 49 Pleurogrammus monopterygius Atka mackerel 320-74 2.0
#> 50 Sebastes alutus Pacific ocean perch 325-76 30.0
#> 51 Pycnopodia helianthoides sunflower sea star 324-73 1.0
#> 52 Atheresthes evermanni Kamchatka flounder 320-74 5.5
#> 53 Sebastes variabilis dusky rockfish 325-76 4.0
#> 54 Zaprora silenus prowfish 325-76 1.0
#> 55 Bathyraja interrupta Bering skate 324-73 1.0
#> 56 Pycnopodia helianthoides sunflower sea star 320-74 1.0
#> 57 Hemilepidotus jordani yellow Irish lord 320-73 1.0
#> 58 Holothuroidea sea cucumber unid. 325-76 3.0
#> 59 Hippoglossoides elassodon flathead sole 320-73 1.0
#> 60 Bathymaster signatus searcher 325-76 2.0
#> 61 Hemilepidotus jordani yellow Irish lord 324-73 1.0
#> 62 Gorgonocephalus eucnemis basketstar 324-73 2.5
#> 63 Thaliacea salp unid. 324-73 5.0
#> 64 Sebastes polyspinis northern rockfish 324-73 1.5
#> 65 Scyphozoa jellyfish unid. 324-73 4.0
#> 66 Modiolus modiolus northern horsemussel 325-76 12.0
#> 67 Pagurus ochotensis Alaskan hermit 324-73 15.0
#> 68 Podothecus accipenserinus sturgeon poacher 324-73 4.0
#> 69 Scyphozoa jellyfish unid. 320-74 1.0
#> 70 Triglops macellus roughspine sculpin 324-73 5.0
#> 71 Pennatuloidea sea whip or sea pen unid. 320-73 NaN
#> 72 Gorgonocephalus eucnemis basketstar 320-74 1.0
#> 73 Crystallichthys cyclospilus blotched snailfish 325-76 1.0
#> 74 Atheresthes evermanni Kamchatka flounder 324-73 3.0
#> 75 Ceramaster patagonicus orange bat sea star 325-76 4.0
#> 76 Pagurus trigonocheirus fuzzy hermit crab 320-74 5.0
#> 77 Actiniaria sea anemone unid. 324-73 2.5
#> 78 Lepidopsetta polyxystra northern rock sole 324-73 1.0
#> 79 Gorgonocephalus eucnemis basketstar 325-76 2.0
#> 80 Elassochirus cavimanus purple hermit 320-74 5.0
#> 81 Bathymaster signatus searcher 324-73 1.0
#> 82 Thaliacea salp unid. 320-74 NaN
#> 83 Pagurus confragosus knobbyhand hermit 324-73 5.0
#> 84 Sarritor frenatus sawback poacher 324-73 3.0
#> 85 Scyphozoa jellyfish unid. 325-76 2.0
#> 86 Modiolus modiolus northern horsemussel 320-74 1.0
#> 87 Elassochirus gilli Pacific red hermit 325-76 2.0
#> 88 Ammodytes sp. sand lance unid. 320-73 2.0
#> 89 Podothecus accipenserinus sturgeon poacher 325-76 1.0
#> 90 Pagurus confragosus knobbyhand hermit 320-74 2.0
#> 91 Pagurus aleuticus Aleutian hermit 324-73 3.0
#> 92 Ceramaster patagonicus orange bat sea star 320-74 1.0
#> 93 Nudibranchia nudibranch unid. 325-76 2.0
#> 94 Gorgonocephalus eucnemis basketstar 320-73 1.0
#> 95 Polychaeta polychaete worm unid. 324-73 NaN
#> 96 Bryozoa bryozoan unid. 320-74 NaN
#> 97 Porifera sponge unid. 324-73 NaN
#> 98 Oregonia gracilis graceful decorator crab 324-73 1.0
#> 99 Nudibranchia nudibranch unid. 324-73 1.0
#> 100 Ophiuroidea brittlestar unid. 320-74 6.0
#> 101 Pagurus brandti sponge hermit 325-76 2.0
#> 102 Rajiformes egg case skate egg case unid. 320-74 4.0
#> 103 Sarritor frenatus sawback poacher 325-76 1.0
#> 104 Actiniaria sea anemone unid. 320-74 1.0
#> 105 Ophiuroidea brittlestar unid. 320-73 5.0
#> 106 Hyas lyratus Pacific lyre crab 320-74 2.0
#> 107 Rajiformes egg case skate egg case unid. 324-73 NaN
#> 108 Crossaster papposus rose sea star 324-73 1.0
#> 109 Pagurus capillatus hairy hermit crab 324-73 1.0
#> 110 Labidochirus splendescens splendid hermit 324-73 1.0
#> 111 Elassochirus tenuimanus widehand hermit crab 325-76 1.0
#> 112 Ophiuroidea brittlestar unid. 325-76 2.0
#> 113 Ascidiacea tunicate unid. 320-74 NaN
#> 114 Ciliatocardium ciliatum hairy cockle 324-73 1.0
#> weight_kg cpue_kgkm2 cpue_nokm2 Freq
#> 1 1211.29 56195.95 74971.85 5
#> 2 322.62 14967.42 0.00 3
#> 3 262.89 10561.90 18058.33 8
#> 4 204.16 8041.73 9827.17 5
#> 5 177.64 7664.13 5324.56 8
#> 6 63.95 2763.53 2999.54 8
#> 7 59.32 2348.62 8125.83 8
#> 8 45.95 2131.78 4082.63 5
#> 9 42.68 1980.07 556.72 8
#> 10 33.60 1447.72 4437.95 6
#> 11 32.02 1383.06 2095.55 7
#> 12 26.93 1113.09 2297.73 6
#> 13 24.43 1089.85 1289.31 7
#> 14 24.25 1077.20 1182.43 7
#> 15 23.05 1069.37 1530.98 8
#> 16 20.68 895.26 763.72 8
#> 17 17.77 749.68 328.39 8
#> 18 18.78 732.43 39.00 1
#> 19 17.56 684.85 1560.02 2
#> 20 16.28 657.13 3437.72 7
#> 21 13.03 604.27 2505.25 6
#> 22 14.62 583.10 440.58 8
#> 23 14.67 574.58 705.01 5
#> 24 11.13 516.36 46.39 4
#> 25 9.35 433.78 788.69 6
#> 26 9.35 433.59 1391.80 1
#> 27 9.08 421.25 231.97 8
#> 28 9.34 403.04 626.43 8
#> 29 9.07 367.07 497.84 6
#> 30 8.89 351.46 158.82 8
#> 31 8.62 351.22 244.47 7
#> 32 7.80 336.97 64.91 4
#> 33 7.00 285.22 244.47 1
#> 34 6.90 271.58 983.99 1
#> 35 5.74 266.30 463.93 5
#> 36 6.39 256.13 1063.12 4
#> 37 5.28 231.61 43.92 2
#> 38 5.56 226.63 40.75 4
#> 39 5.43 222.48 412.45 4
#> 40 4.98 202.11 263.46 2
#> 41 4.92 194.71 318.42 5
#> 42 4.32 186.14 430.87 1
#> 43 3.58 140.91 39.36 1
#> 44 3.42 138.24 40.05 3
#> 45 2.91 135.19 185.57 7
#> 46 2.72 126.19 46.39 3
#> 47 2.50 108.88 130.09 5
#> 48 2.26 104.80 371.15 7
#> 49 2.60 104.33 81.00 5
#> 50 2.16 100.16 1391.80 2
#> 51 2.50 98.56 39.36 2
#> 52 2.31 95.30 240.15 4
#> 53 1.63 75.71 185.57 1
#> 54 1.59 73.86 46.39 1
#> 55 1.85 72.82 39.36 1
#> 56 1.32 59.41 45.01 2
#> 57 1.33 57.39 43.09 6
#> 58 0.99 46.12 139.18 1
#> 59 0.95 40.76 43.09 7
#> 60 0.76 35.07 92.79 2
#> 61 0.88 34.64 39.36 6
#> 62 0.81 32.54 101.17 5
#> 63 0.76 30.73 98.40 4
#> 64 0.59 23.52 59.73 5
#> 65 0.58 22.67 157.44 3
#> 66 0.38 17.40 556.72 2
#> 67 0.44 17.40 590.40 1
#> 68 0.36 14.67 162.98 2
#> 69 0.30 11.51 39.00 3
#> 70 0.29 11.49 196.80 1
#> 71 0.26 11.12 0.00 1
#> 72 0.24 10.62 45.01 5
#> 73 0.22 10.30 46.39 1
#> 74 0.23 9.30 121.54 4
#> 75 0.20 9.28 185.57 2
#> 76 0.19 8.45 227.07 1
#> 77 0.21 8.08 99.09 3
#> 78 0.17 6.85 39.36 6
#> 79 0.14 6.73 92.79 5
#> 80 0.15 6.72 227.07 1
#> 81 0.15 6.19 40.75 2
#> 82 0.13 5.89 0.00 4
#> 83 0.14 5.79 203.73 2
#> 84 0.14 5.51 118.08 2
#> 85 0.10 4.41 92.79 3
#> 86 0.09 4.09 45.41 2
#> 87 0.09 3.94 92.79 1
#> 88 0.08 3.45 86.17 1
#> 89 0.07 3.34 46.39 2
#> 90 0.06 2.72 90.83 2
#> 91 0.07 2.69 122.24 1
#> 92 0.06 2.63 45.41 2
#> 93 0.06 2.55 92.79 2
#> 94 0.06 2.41 43.09 5
#> 95 0.05 2.20 0.00 1
#> 96 0.04 2.00 0.00 1
#> 97 0.05 1.87 0.00 3
#> 98 0.04 1.65 39.36 1
#> 99 0.04 1.47 40.75 2
#> 100 0.03 1.44 270.04 3
#> 101 0.03 1.39 92.79 1
#> 102 0.03 1.17 90.01 3
#> 103 0.02 1.11 46.39 2
#> 104 0.02 0.91 45.41 3
#> 105 0.02 0.86 215.43 3
#> 106 0.02 0.82 90.83 1
#> 107 0.02 0.81 0.00 3
#> 108 0.01 0.55 39.36 1
#> 109 0.01 0.47 39.36 1
#> 110 0.01 0.33 40.75 1
#> 111 0.00 0.23 46.39 1
#> 112 0.00 0.23 92.79 3
#> 113 0.00 0.09 0.00 1
#> 114 0.00 0.08 39.36 1
#>
#> $haul
#> year station haul stratum vessel_name date_time
#> 1 2016 320-73 3 721 ALASKA PROVIDER 2016-06-07 15:15:11
#> 2 2016 320-74 5 721 SEA STORM 2016-06-07 18:04:38
#> 3 2016 320-74 7 722 SEA STORM 2016-06-08 09:23:26
#> 4 2016 324-73 4 721 SEA STORM 2016-06-07 16:05:45
#> 5 2018 320-73 10 721 SEA STORM 2018-06-11 17:28:48
#> 6 2018 320-74 9 722 SEA STORM 2018-06-11 15:08:22
#> 7 2018 324-73 1 721 OCEAN EXPLORER 2018-06-09 07:27:06
#> 8 2018 325-76 2 721 OCEAN EXPLORER 2018-06-09 11:58:02
#> latitude_dd_start longitude_dd_start bottom_temperature_c
#> 1 54.20566 -166.0491 6.3
#> 2 54.22642 -166.0167 6.4
#> 3 54.25784 -166.0004 6.2
#> 4 54.21666 -165.7556 6.4
#> 5 54.21682 -166.0323 5.6
#> 6 54.24662 -166.0222 4.5
#> 7 54.21011 -165.7440 5.3
#> 8 54.35293 -165.5989 5.0
#> surface_temperature_c depth_m distance_fished_km net_width_m net_height_m
#> 1 7.1 61 1.526 15.209 5.907
#> 2 7.4 74 1.515 14.666 7.647
#> 3 6.9 110 1.441 15.281 7.178
#> 4 7.6 93 1.436 17.091 6.704
#> 5 5.8 63 1.436 15.560 6.568
#> 6 7.0 109 1.451 17.671 6.146
#> 7 NA 96 1.549 16.402 4.755
#> 8 6.2 83 1.321 16.317 5.575
#> area_swept_km2 duration_hr total_weight_kg
#> 1 0.02320893 0.272 590.05
#> 2 0.02221899 0.274 410.23
#> 3 0.02201992 0.265 410.23
#> 4 0.02454268 0.269 111.26
#> 5 0.02234416 0.294 32.12
#> 6 0.02564062 0.269 867.17
#> 7 0.02540670 0.274 571.17
#> 8 0.02155476 0.239 1735.27
#>
## for default 10 years and nearby stations for all species (a typical use-case) ----
get_catch_haul_history(
survey = "AI",
years = NA, # default
station = "324-73",
grid_buffer = 3)
#> $catch
#> $catch$`2002`
#> haul scientific_name common_name station count
#> 681 23 Gadus chalcogrammus walleye pollock 321-74 4153
#> 682 23 Lepidopsetta bilineata southern rock sole 321-74 176
#> 683 28 Hippoglossus stenolepis Pacific halibut 320-74 49
#> 684 28 Gadus macrocephalus Pacific cod 320-74 41
#> 685 23 Atheresthes stomias arrowtooth flounder 321-74 85
#> 686 16 Gadus chalcogrammus walleye pollock 324-73 50
#> 687 28 Lepidopsetta bilineata southern rock sole 320-74 63
#> 688 27 Lepidopsetta bilineata southern rock sole 320-73 25
#> 689 16 Atheresthes stomias arrowtooth flounder 324-73 154
#> 690 23 Hippoglossus stenolepis Pacific halibut 321-74 13
#> 691 23 Gadus macrocephalus Pacific cod 321-74 15
#> 692 16 Somniosus pacificus Pacific sleeper shark 324-73 1
#> 693 28 Lepidopsetta polyxystra northern rock sole 320-74 50
#> 694 16 Gadus macrocephalus Pacific cod 324-73 15
#> 695 16 Glyptocephalus zachirus rex sole 324-73 47
#> 696 28 Atheresthes stomias arrowtooth flounder 320-74 47
#> 697 27 Hippoglossus stenolepis Pacific halibut 320-73 16
#> 698 16 Hippoglossoides elassodon flathead sole 324-73 85
#> 699 16 Hippoglossus stenolepis Pacific halibut 324-73 3
#> 700 27 Atheresthes stomias arrowtooth flounder 320-73 38
#> 701 23 Lepidopsetta polyxystra northern rock sole 321-74 10
#> 702 28 Hemilepidotus jordani yellow Irish lord 320-74 8
#> 703 16 Chionoecetes bairdi Tanner crab 324-73 9
#> 704 27 Myoxocephalus polyacanthocephalus great sculpin 320-73 1
#> 705 28 Myoxocephalus polyacanthocephalus great sculpin 320-74 1
#> 706 27 Lepidopsetta polyxystra northern rock sole 320-73 7
#> 707 27 Gadus macrocephalus Pacific cod 320-73 2
#> 708 28 Actiniaria sea anemone unid. 320-74 13
#> 709 16 Lepidopsetta bilineata southern rock sole 324-73 2
#> 710 28 Scyphozoa jellyfish unid. 320-74 3
#> 711 27 Hemilepidotus jordani yellow Irish lord 320-73 3
#> 712 28 Sebastes variabilis dusky rockfish 320-74 2
#> 713 27 Gadus chalcogrammus walleye pollock 320-73 3
#> 714 16 Myoxocephalus polyacanthocephalus great sculpin 324-73 1
#> 715 28 Hexagrammos decagrammus kelp greenling 320-74 2
#> 716 23 Sebastes ciliatus dark rockfish 321-74 1
#> 717 27 Pleurogrammus monopterygius Atka mackerel 320-73 3
#> 718 27 Scyphozoa jellyfish unid. 320-73 3
#> 719 28 Porifera sponge unid. 320-74 NA
#> 720 23 Limanda aspera yellowfin sole 321-74 1
#> 721 23 Hippoglossoides elassodon flathead sole 321-74 2
#> 722 23 Pleurogrammus monopterygius Atka mackerel 321-74 2
#> 723 16 Podothecus accipenserinus sturgeon poacher 324-73 14
#> 724 27 Lethasterias nanimensis blackspined sea star 320-73 3
#> 725 16 Porifera sponge unid. 324-73 1
#> 726 16 Lepidopsetta polyxystra northern rock sole 324-73 1
#> 727 16 Scyphozoa jellyfish unid. 324-73 1
#> 728 16 Hemilepidotus jordani yellow Irish lord 324-73 1
#> 729 16 Sebastes variabilis dusky rockfish 324-73 1
#> 730 23 Gymnocanthus galeatus armorhead sculpin 321-74 1
#> 731 16 Sebastes polyspinis northern rockfish 324-73 1
#> 732 23 Echinarachnius parma parma sand dollar 321-74 4
#> 733 28 Thaliacea salp unid. 320-74 NA
#> 734 16 Polyplacophora chiton unid. 324-73 1
#> 735 16 Ascidiacea tunicate unid. 324-73 NA
#> 736 27 Podothecus accipenserinus sturgeon poacher 320-73 1
#> 737 23 Podothecus accipenserinus sturgeon poacher 321-74 1
#> 738 28 Ammodytes sp. sand lance unid. 320-74 1
#> 739 28 Holothuroidea sea cucumber unid. 320-74 1
#> 740 27 Ophiuroidea brittlestar unid. 320-73 1
#> 741 27 Porifera sponge unid. 320-73 NA
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 681 3769.262 1.706609e+05 188035.45734
#> 682 171.600 7.769536e+03 7968.75523
#> 683 64.400 3.002039e+03 2284.16036
#> 684 56.900 2.652423e+03 1911.23622
#> 685 49.000 2.218574e+03 3848.54656
#> 686 45.400 1.950869e+03 2148.53465
#> 687 35.900 1.673497e+03 2936.77761
#> 688 30.300 1.584891e+03 1307.66570
#> 689 29.800 1.280527e+03 6617.48671
#> 690 27.204 1.231716e+03 588.60124
#> 691 27.100 1.227007e+03 679.15528
#> 692 25.800 1.108644e+03 42.97069
#> 693 25.200 1.174711e+03 2330.77588
#> 694 19.100 8.207402e+02 644.56039
#> 695 17.500 7.519871e+02 2019.62257
#> 696 17.200 8.017869e+02 2190.92933
#> 697 16.832 8.804252e+02 836.90605
#> 698 16.800 7.219076e+02 3652.50890
#> 699 16.774 7.207904e+02 128.91208
#> 700 13.900 7.270621e+02 1987.65187
#> 701 6.300 2.852452e+02 452.77018
#> 702 5.796 2.701835e+02 372.92414
#> 703 5.086 2.185489e+02 386.73624
#> 704 4.456 2.330783e+02 52.30663
#> 705 2.780 1.295911e+02 46.61552
#> 706 2.682 1.402864e+02 366.14640
#> 707 2.574 1.346373e+02 104.61326
#> 708 2.102 9.798582e+01 606.00173
#> 709 2.102 9.032440e+01 85.94139
#> 710 1.902 8.866272e+01 139.84655
#> 711 1.846 9.655804e+01 156.91988
#> 712 1.836 8.558609e+01 93.23104
#> 713 1.638 8.567826e+01 156.91988
#> 714 1.458 6.265127e+01 42.97069
#> 715 1.442 6.721958e+01 93.23104
#> 716 1.326 6.003733e+01 45.27702
#> 717 1.308 6.841707e+01 156.91988
#> 718 1.308 6.841707e+01 156.91988
#> 719 1.132 5.276877e+01 0.00000
#> 720 0.996 4.509591e+01 45.27702
#> 721 0.962 4.355649e+01 90.55404
#> 722 0.920 4.165486e+01 90.55404
#> 723 0.904 3.884551e+01 601.58970
#> 724 0.786 4.111301e+01 156.91988
#> 725 0.543 2.333309e+01 42.97069
#> 726 0.406 1.744610e+01 42.97069
#> 727 0.332 1.426627e+01 42.97069
#> 728 0.312 1.340686e+01 42.97069
#> 729 0.294 1.263338e+01 42.97069
#> 730 0.150 6.791553e+00 45.27702
#> 731 0.140 6.015897e+00 42.97069
#> 732 0.126 5.704904e+00 181.10807
#> 733 0.116 5.407400e+00 0.00000
#> 734 0.090 3.867362e+00 42.97069
#> 735 0.080 3.437655e+00 0.00000
#> 736 0.056 2.929171e+00 52.30663
#> 737 0.054 2.444959e+00 45.27702
#> 738 0.020 9.323100e-01 46.61552
#> 739 0.016 7.458480e-01 46.61552
#> 740 0.010 5.230660e-01 52.30663
#> 741 0.004 2.092270e-01 0.00000
#>
#> $catch$`2004`
#> haul scientific_name common_name station count
#> 605 4 Pleurogrammus monopterygius Atka mackerel 326-76 10363
#> 606 5 Gadus chalcogrammus walleye pollock 324-73 777
#> 607 7 Gadus macrocephalus Pacific cod 320-74 96
#> 608 1 Atheresthes stomias arrowtooth flounder 321-74 346
#> 609 7 Hippoglossus stenolepis Pacific halibut 320-74 107
#> 610 6 Lepidopsetta bilineata southern rock sole 320-73 127
#> 611 6 Lepidopsetta polyxystra northern rock sole 320-73 217
#> 612 4 Gadus macrocephalus Pacific cod 326-76 12
#> 613 7 Lepidopsetta bilineata southern rock sole 320-74 93
#> 614 5 Atheresthes stomias arrowtooth flounder 324-73 240
#> 615 1 Lepidopsetta bilineata southern rock sole 321-74 91
#> 616 4 Porifera sponge unid. 326-76 1
#> 617 4 Hippoglossus stenolepis Pacific halibut 326-76 9
#> 618 5 Hippoglossoides elassodon flathead sole 324-73 237
#> 619 7 Lepidopsetta polyxystra northern rock sole 320-74 74
#> 620 1 Gadus macrocephalus Pacific cod 321-74 25
#> 621 6 Hippoglossus stenolepis Pacific halibut 320-73 22
#> 622 1 Lepidopsetta polyxystra northern rock sole 321-74 50
#> 623 7 Pleurogrammus monopterygius Atka mackerel 320-74 17
#> 624 1 Hippoglossus stenolepis Pacific halibut 321-74 7
#> 625 5 Glyptocephalus zachirus rex sole 324-73 49
#> 626 5 Beringraja binoculata big skate 324-73 1
#> 627 5 Hippoglossus stenolepis Pacific halibut 324-73 8
#> 628 6 Gadus macrocephalus Pacific cod 320-73 9
#> 629 6 Atheresthes stomias arrowtooth flounder 320-73 24
#> 630 1 Hippoglossoides elassodon flathead sole 321-74 14
#> 631 5 Gadus macrocephalus Pacific cod 324-73 5
#> 632 5 Arctoraja parmifera Alaska skate 324-73 1
#> 633 6 Pleurogrammus monopterygius Atka mackerel 320-73 9
#> 634 5 Myoxocephalus polyacanthocephalus great sculpin 324-73 3
#> 635 5 Hemilepidotus jordani yellow Irish lord 324-73 6
#> 636 1 Hemilepidotus jordani yellow Irish lord 321-74 3
#> 637 5 Pleurogrammus monopterygius Atka mackerel 324-73 3
#> 638 7 Hemilepidotus jordani yellow Irish lord 320-74 3
#> 639 4 Hemitripterus bolini bigmouth sculpin 326-76 1
#> 640 5 Pleuronectes quadrituberculatus Alaska plaice 324-73 1
#> 641 1 Pleurogrammus monopterygius Atka mackerel 321-74 2
#> 642 7 Hexagrammos decagrammus kelp greenling 320-74 4
#> 643 7 Gadus chalcogrammus walleye pollock 320-74 2
#> 644 6 Aptocyclus ventricosus smooth lumpsucker 320-73 1
#> 645 1 Sebastes variabilis dusky rockfish 321-74 1
#> 646 5 Podothecus accipenserinus sturgeon poacher 324-73 14
#> 647 5 Lepidopsetta bilineata southern rock sole 324-73 2
#> 648 6 Gadus chalcogrammus walleye pollock 320-73 1
#> 649 4 Sebastes alutus Pacific ocean perch 326-76 4
#> 650 1 Thaliacea salp unid. 321-74 NA
#> 651 5 Chionoecetes bairdi Tanner crab 324-73 4
#> 652 5 Scyphozoa jellyfish unid. 324-73 2
#> 653 5 Gymnocanthus galeatus armorhead sculpin 324-73 2
#> 654 4 Sebastes polyspinis northern rockfish 326-76 1
#> 655 5 Clupea pallasii Pacific herring 324-73 1
#> 656 4 Sebastes variegatus harlequin rockfish 326-76 1
#> 657 6 Thaliacea salp unid. 320-73 NA
#> 658 5 Anoplopoma fimbria sablefish 324-73 1
#> 659 5 Porifera sponge unid. 324-73 NA
#> 660 1 Elassochirus cavimanus purple hermit 321-74 4
#> 661 5 Microstomus pacificus Dover sole 324-73 1
#> 662 1 Podothecus accipenserinus sturgeon poacher 321-74 2
#> 663 6 Scyphozoa jellyfish unid. 320-73 NA
#> 664 1 Pagurus ochotensis Alaskan hermit 321-74 2
#> 665 1 Ascidiacea tunicate unid. 321-74 NA
#> 666 1 Scyphozoa jellyfish unid. 321-74 1
#> 667 7 Modiolus modiolus northern horsemussel 320-74 1
#> 668 5 Pagurus aleuticus Aleutian hermit 324-73 2
#> 669 1 Elassochirus tenuimanus widehand hermit crab 321-74 2
#> 670 5 Crossaster papposus rose sea star 324-73 1
#> 671 7 Elassochirus tenuimanus widehand hermit crab 320-74 3
#> 672 7 Ammodytes sp. sand lance unid. 320-74 1
#> 673 1 Hyas lyratus Pacific lyre crab 321-74 1
#> 674 1 Porifera sponge unid. 321-74 NA
#> 675 7 Ophiuroidea brittlestar unid. 320-74 1
#> 676 1 Ophiuroidea brittlestar unid. 321-74 2
#> 677 4 Pagurus dalli whiteknee hermit 326-76 1
#> 678 1 Actiniaria sea anemone unid. 321-74 1
#> 679 1 Pagurus brandti sponge hermit 321-74 1
#> 680 5 Polychaeta polychaete worm unid. 324-73 1
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 605 11614.408 6.412954e+05 572198.24397
#> 606 804.260 3.108126e+04 30027.77395
#> 607 243.080 1.084801e+04 4284.22246
#> 608 184.880 6.666225e+03 12475.73542
#> 609 167.294 7.465882e+03 4775.12295
#> 610 120.050 5.364186e+03 5674.73238
#> 611 97.040 4.336032e+03 9696.19628
#> 612 69.470 3.835821e+03 662.58602
#> 613 65.860 2.939155e+03 4150.34051
#> 614 61.490 2.376329e+03 9274.98809
#> 615 57.400 2.069674e+03 3281.19053
#> 616 52.944 2.923330e+03 55.21550
#> 617 52.182 2.881255e+03 496.93951
#> 618 45.630 1.763407e+03 9159.05074
#> 619 36.060 1.609261e+03 3302.42148
#> 620 35.300 1.272813e+03 901.42597
#> 621 22.307 9.967422e+02 983.02451
#> 622 21.050 7.590007e+02 1802.85194
#> 623 21.030 9.385125e+02 758.66439
#> 624 19.555 7.050954e+02 252.39927
#> 625 16.580 6.407471e+02 1893.64340
#> 626 15.260 5.897347e+02 38.64578
#> 627 12.636 4.883281e+02 309.16627
#> 628 12.610 5.634518e+02 402.14639
#> 629 10.670 4.767669e+02 1072.39037
#> 630 9.380 3.382150e+02 504.79854
#> 631 7.010 2.709069e+02 193.22892
#> 632 6.560 2.535163e+02 38.64578
#> 633 4.782 2.136738e+02 402.14639
#> 634 4.528 1.749881e+02 115.93735
#> 635 4.020 1.553561e+02 231.87470
#> 636 3.562 1.284352e+02 108.17112
#> 637 2.530 9.777383e+01 115.93735
#> 638 2.159 9.635038e+01 133.88195
#> 639 2.100 1.159526e+02 55.21550
#> 640 2.034 7.860552e+01 38.64578
#> 641 1.666 6.007103e+01 72.11408
#> 642 1.526 6.810129e+01 178.50927
#> 643 1.472 6.569141e+01 89.25463
#> 644 1.414 6.318167e+01 44.68293
#> 645 1.282 4.622512e+01 36.05704
#> 646 1.092 4.220120e+01 541.04097
#> 647 1.052 4.065536e+01 77.29157
#> 648 0.928 4.146576e+01 44.68293
#> 649 0.886 4.892093e+01 220.86201
#> 650 0.846 3.050426e+01 0.00000
#> 651 0.800 3.091663e+01 154.58313
#> 652 0.640 2.473330e+01 77.29157
#> 653 0.490 1.893643e+01 77.29157
#> 654 0.442 2.440525e+01 55.21550
#> 655 0.366 1.414436e+01 38.64578
#> 656 0.324 1.788982e+01 55.21550
#> 657 0.286 1.277932e+01 0.00000
#> 658 0.240 9.274988e+00 38.64578
#> 659 0.230 8.888530e+00 0.00000
#> 660 0.228 8.221005e+00 144.22815
#> 661 0.194 7.497282e+00 38.64578
#> 662 0.164 5.913354e+00 72.11408
#> 663 0.132 5.898147e+00 0.00000
#> 664 0.120 4.326845e+00 72.11408
#> 665 0.116 4.182616e+00 0.00000
#> 666 0.108 3.894160e+00 36.05704
#> 667 0.080 3.570185e+00 44.62732
#> 668 0.076 2.937080e+00 77.29157
#> 669 0.072 2.596107e+00 72.11408
#> 670 0.050 1.932289e+00 38.64578
#> 671 0.044 1.963602e+00 133.88195
#> 672 0.030 1.338820e+00 44.62732
#> 673 0.018 6.490270e-01 36.05704
#> 674 0.010 3.605700e-01 0.00000
#> 675 0.006 2.677640e-01 44.62732
#> 676 0.006 2.163420e-01 72.11408
#> 677 0.006 3.312930e-01 55.21550
#> 678 0.002 7.211400e-02 36.05704
#> 679 0.002 7.211400e-02 36.05704
#> 680 0.001 3.864600e-02 38.64578
#>
#> $catch$`2006`
#> haul scientific_name common_name station
#> 493 7 Atheresthes stomias arrowtooth flounder 320-74
#> 494 5 Porifera sponge unid. 325-76
#> 495 6 Gadus chalcogrammus walleye pollock 324-73
#> 496 7 Atheresthes stomias arrowtooth flounder 321-74
#> 497 6 Atheresthes stomias arrowtooth flounder 324-73
#> 498 5 Hemilepidotus jordani yellow Irish lord 325-76
#> 499 7 Gadus chalcogrammus walleye pollock 321-74
#> 500 5 Lepidopsetta polyxystra northern rock sole 325-76
#> 501 7 Hippoglossus stenolepis Pacific halibut 320-74
#> 502 7 Lepidopsetta polyxystra northern rock sole 320-74
#> 503 5 Hippoglossus stenolepis Pacific halibut 325-76
#> 504 7 Gadus macrocephalus Pacific cod 321-74
#> 505 7 Lepidopsetta bilineata southern rock sole 321-74
#> 506 7 Hippoglossus stenolepis Pacific halibut 321-74
#> 507 6 Hippoglossus stenolepis Pacific halibut 324-73
#> 508 5 Gadus macrocephalus Pacific cod 325-76
#> 509 6 Enteroctopus dofleini giant octopus 324-73
#> 510 6 Hippoglossoides elassodon flathead sole 324-73
#> 511 7 Hemilepidotus jordani yellow Irish lord 320-74
#> 512 7 Glyptocephalus zachirus rex sole 320-74
#> 513 5 Pleurogrammus monopterygius Atka mackerel 325-76
#> 514 6 Gadus macrocephalus Pacific cod 324-73
#> 515 5 Atheresthes stomias arrowtooth flounder 325-76
#> 516 5 Lepidopsetta bilineata southern rock sole 325-76
#> 517 7 Lepidopsetta polyxystra northern rock sole 321-74
#> 518 5 Gadus chalcogrammus walleye pollock 325-76
#> 519 7 Hippoglossoides elassodon flathead sole 321-74
#> 520 5 Arctoraja parmifera Alaska skate 325-76
#> 521 6 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 522 7 Myoxocephalus polyacanthocephalus great sculpin 320-74
#> 523 6 Glyptocephalus zachirus rex sole 324-73
#> 524 5 Bathymaster signatus searcher 325-76
#> 525 7 Gadus macrocephalus Pacific cod 320-74
#> 526 7 Gadus chalcogrammus walleye pollock 320-74
#> 527 5 Hippoglossoides elassodon flathead sole 325-76
#> 528 5 Hexagrammos decagrammus kelp greenling 325-76
#> 529 7 Pleurogrammus monopterygius Atka mackerel 321-74
#> 530 7 Actiniaria sea anemone unid. 321-74
#> 531 5 Oncorhynchus keta chum salmon 325-76
#> 532 7 Hemilepidotus jordani yellow Irish lord 321-74
#> 533 7 Lepidopsetta bilineata southern rock sole 320-74
#> 534 5 Lethasterias nanimensis blackspined sea star 325-76
#> 535 5 Zoanthidae sp. A hot dog zoanthid 325-76
#> 536 5 Holothuroidea sea cucumber unid. 325-76
#> 537 5 Pagurus brandti sponge hermit 325-76
#> 538 6 Lepidopsetta bilineata southern rock sole 324-73
#> 539 7 Glyptocephalus zachirus rex sole 321-74
#> 540 6 Lepidopsetta polyxystra northern rock sole 324-73
#> 541 5 Sebastes alutus Pacific ocean perch 325-76
#> 542 6 Chionoecetes bairdi Tanner crab 324-73
#> 543 7 Gorgonocephalus eucnemis basketstar 321-74
#> 544 5 Gymnocanthus galeatus armorhead sculpin 325-76
#> 545 7 Atheresthes evermanni Kamchatka flounder 320-74
#> 546 5 Actiniaria sea anemone unid. 325-76
#> 547 6 Gorgonocephalus eucnemis basketstar 324-73
#> 548 6 Pagurus trigonocheirus fuzzy hermit crab 324-73
#> 549 6 Hemilepidotus jordani yellow Irish lord 324-73
#> 550 6 Thaliacea salp unid. 324-73
#> 551 6 Lethasterias nanimensis blackspined sea star 324-73
#> 552 5 Crossaster papposus rose sea star 325-76
#> 553 7 Chionoecetes bairdi Tanner crab 321-74
#> 554 5 Modiolus modiolus northern horsemussel 325-76
#> 555 7 Gymnocanthus galeatus armorhead sculpin 321-74
#> 556 5 Mediaster aequalis vermilion sea star 325-76
#> 557 5 Sebastes ciliatus dark rockfish 325-76
#> 558 6 Podothecus accipenserinus sturgeon poacher 324-73
#> 559 6 Gymnocanthus galeatus armorhead sculpin 324-73
#> 560 5 Elassochirus cavimanus purple hermit 325-76
#> 561 7 Pagurus ochotensis Alaskan hermit 321-74
#> 562 5 Cheiraster dawsoni fragile sea star 325-76
#> 563 5 Ceramaster japonicus red bat star 325-76
#> 564 5 Triglops forficatus scissortail sculpin 325-76
#> 565 6 Erimacrus isenbeckii horsehair crab 324-73
#> 566 5 Acantholithodes hispidus fuzzy crab 325-76
#> 567 7 Holothuroidea sea cucumber unid. 320-74
#> 568 5 Scyphozoa jellyfish unid. 325-76
#> 569 7 Podothecus accipenserinus sturgeon poacher 321-74
#> 570 7 Triglops macellus roughspine sculpin 321-74
#> 571 6 Pagurus aleuticus Aleutian hermit 324-73
#> 572 6 Crossaster papposus rose sea star 324-73
#> 573 7 Lethasterias nanimensis blackspined sea star 321-74
#> 574 5 Pseudarchaster parelii scarlet sea star 325-76
#> 575 5 Ascidiacea tunicate unid. 325-76
#> 576 6 Scyphozoa jellyfish unid. 324-73
#> 577 7 Elassochirus tenuimanus widehand hermit crab 321-74
#> 578 7 Hyas lyratus Pacific lyre crab 321-74
#> 579 5 Polychaeta polychaete worm unid. 325-76
#> 580 5 Pagurus aleuticus Aleutian hermit 325-76
#> 581 7 Crossaster papposus rose sea star 321-74
#> 582 6 Pseudarchaster parelii scarlet sea star 324-73
#> 583 5 Rhinolithodes wosnessenskii rhinoceros crab 325-76
#> 584 7 Hyas lyratus Pacific lyre crab 320-74
#> 585 7 Pagurus aleuticus Aleutian hermit 320-74
#> 586 7 Elassochirus cavimanus purple hermit 320-74
#> 587 5 Elassochirus tenuimanus widehand hermit crab 325-76
#> 588 7 Modiolus modiolus northern horsemussel 321-74
#> 589 7 Pseudarchaster parelii scarlet sea star 321-74
#> 590 7 Ascidiacea tunicate unid. 321-74
#> 591 7 Actiniaria sea anemone unid. 320-74
#> 592 5 Leptychaster arcticus North Pacific sea star 325-76
#> 593 5 Bryozoa bryozoan unid. 325-76
#> 594 7 Porifera sponge unid. 320-74
#> 595 5 Ophiuroidea brittlestar unid. 325-76
#> 596 7 Ctenodiscus crispatus common mud star 321-74
#> 597 6 Cheiraster dawsoni fragile sea star 324-73
#> 598 5 Pedicellaster magister majestic sea star 325-76
#> 599 7 Ophiuroidea brittlestar unid. 321-74
#> 600 6 Pagurus rathbuni longfinger hermit 324-73
#> 601 5 Leptasterias hylodes Aleutian sea star 325-76
#> 602 7 Pagurus brandti sponge hermit 320-74
#> 603 5 Oregonia gracilis graceful decorator crab 325-76
#> 604 5 Nudibranchia nudibranch unid. 325-76
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 493 438 417.490 18447.288758 19353.54733
#> 494 NA 346.596 15928.687189 0.00000
#> 495 300 251.768 10546.830065 12567.31999
#> 496 220 114.970 5388.772492 10311.64607
#> 497 242 52.480 2198.443177 10137.63813
#> 498 51 42.162 1937.660300 2343.83272
#> 499 48 41.520 1946.088840 2249.81369
#> 500 112 38.202 1755.668582 5147.24049
#> 501 19 37.129 1640.588719 839.53744
#> 502 64 35.440 1565.958259 2827.91559
#> 503 10 34.638 1591.876037 459.57504
#> 504 9 28.700 1345.201101 421.84007
#> 505 52 28.260 1324.577808 2437.29816
#> 506 10 26.350 1235.053972 468.71119
#> 507 11 23.507 984.733303 460.80173
#> 508 12 21.200 974.299093 551.49005
#> 509 1 21.130 885.158238 41.89107
#> 510 97 20.770 870.077454 4063.43346
#> 511 26 17.460 771.490722 1148.84071
#> 512 25 17.320 765.304657 1104.65453
#> 513 15 14.700 675.575314 689.36257
#> 514 10 13.102 548.856755 418.91067
#> 515 35 10.780 495.421897 1608.51265
#> 516 13 8.326 382.642181 597.44756
#> 517 25 8.210 384.811883 1171.77796
#> 518 6 7.160 329.055731 275.74503
#> 519 16 6.068 284.413947 749.93790
#> 520 1 5.980 274.825876 45.95750
#> 521 1 5.930 248.414025 41.89107
#> 522 2 5.630 248.768200 88.37236
#> 523 22 5.500 230.400867 921.60347
#> 524 18 4.740 217.838571 827.23508
#> 525 3 4.310 190.442441 132.55854
#> 526 4 4.240 187.349408 176.74472
#> 527 7 4.112 188.977258 321.70253
#> 528 9 3.600 165.447016 413.61754
#> 529 3 3.530 165.455048 140.61336
#> 530 24 3.490 163.580204 1124.90684
#> 531 1 3.120 143.387414 45.95750
#> 532 2 2.410 112.959396 93.74224
#> 533 2 1.820 80.418850 88.37236
#> 534 10 1.348 61.950716 459.57504
#> 535 NA 1.230 56.527730 0.00000
#> 536 2 1.170 53.770280 91.91501
#> 537 29 1.146 52.667300 1332.76763
#> 538 1 1.118 46.834213 41.89107
#> 539 1 0.930 43.590140 46.87112
#> 540 2 0.902 37.785742 83.78213
#> 541 7 0.814 37.409409 321.70253
#> 542 12 0.594 24.883294 502.69280
#> 543 6 0.566 26.529053 281.22671
#> 544 2 0.552 25.368542 91.91501
#> 545 1 0.550 24.302400 44.18618
#> 546 19 0.454 20.864707 873.19258
#> 547 3 0.364 15.248348 125.67320
#> 548 1 0.360 15.080784 41.89107
#> 549 1 0.348 14.578091 41.89107
#> 550 NA 0.338 14.159181 0.00000
#> 551 3 0.336 14.075398 125.67320
#> 552 8 0.314 14.430656 367.66003
#> 553 2 0.296 13.873851 93.74224
#> 554 9 0.270 12.408526 413.61754
#> 555 2 0.264 12.373975 93.74224
#> 556 4 0.232 10.662141 183.83002
#> 557 1 0.226 10.386396 45.95750
#> 558 3 0.222 9.299817 125.67320
#> 559 1 0.184 7.707956 41.89107
#> 560 5 0.178 8.180436 229.78752
#> 561 3 0.170 7.968090 140.61336
#> 562 11 0.168 7.720861 505.53255
#> 563 3 0.168 7.720861 137.87251
#> 564 5 0.156 7.169371 229.78752
#> 565 1 0.146 6.116096 41.89107
#> 566 4 0.136 6.250221 183.83002
#> 567 3 0.096 4.241873 132.55854
#> 568 NA 0.086 3.952345 0.00000
#> 569 1 0.072 3.374721 46.87112
#> 570 1 0.066 3.093494 46.87112
#> 571 4 0.064 2.681028 167.56427
#> 572 1 0.062 2.597246 41.89107
#> 573 1 0.058 2.718525 46.87112
#> 574 2 0.058 2.665535 91.91501
#> 575 1 0.058 2.665535 45.95750
#> 576 1 0.054 2.262118 41.89107
#> 577 1 0.048 2.249814 46.87112
#> 578 1 0.044 2.062329 46.87112
#> 579 NA 0.042 1.930215 0.00000
#> 580 2 0.038 1.746385 91.91501
#> 581 1 0.036 1.687360 46.87112
#> 582 1 0.036 1.508078 41.89107
#> 583 1 0.034 1.562555 45.95750
#> 584 1 0.030 1.325585 44.18618
#> 585 1 0.028 1.237213 44.18618
#> 586 1 0.028 1.237213 44.18618
#> 587 1 0.024 1.102980 45.95750
#> 588 1 0.022 1.031165 46.87112
#> 589 1 0.020 0.937422 46.87112
#> 590 1 0.020 0.937422 46.87112
#> 591 1 0.018 0.795351 44.18618
#> 592 1 0.016 0.735320 45.95750
#> 593 NA 0.014 0.643405 0.00000
#> 594 1 0.012 0.530234 44.18618
#> 595 4 0.012 0.551490 183.83002
#> 596 1 0.010 0.468711 46.87112
#> 597 1 0.010 0.418911 41.89107
#> 598 2 0.010 0.459575 91.91501
#> 599 2 0.008 0.374969 93.74224
#> 600 1 0.008 0.335129 41.89107
#> 601 2 0.006 0.275745 91.91501
#> 602 1 0.002 0.088372 44.18618
#> 603 1 0.002 0.091915 45.95750
#> 604 1 0.002 0.091915 45.95750
#>
#> $catch$`2010`
#> haul scientific_name common_name station
#> 417 7 Gadus chalcogrammus walleye pollock 325-76
#> 418 4 Pleurogrammus monopterygius Atka mackerel 326-76
#> 419 3 Pleurogrammus monopterygius Atka mackerel 326-76
#> 420 3 Gadus chalcogrammus walleye pollock 326-76
#> 421 3 Gadus chalcogrammus walleye pollock 324-73
#> 422 5 Lepidopsetta polyxystra northern rock sole 320-73
#> 423 7 Gadus macrocephalus Pacific cod 325-76
#> 424 5 Lepidopsetta bilineata southern rock sole 320-73
#> 425 7 Lepidopsetta polyxystra northern rock sole 325-76
#> 426 7 Hippoglossus stenolepis Pacific halibut 325-76
#> 427 3 Gadus macrocephalus Pacific cod 326-76
#> 428 3 Atheresthes stomias arrowtooth flounder 326-76
#> 429 7 Hippoglossoides elassodon flathead sole 325-76
#> 430 7 Pleurogrammus monopterygius Atka mackerel 325-76
#> 431 5 Hippoglossus stenolepis Pacific halibut 320-73
#> 432 3 Atheresthes stomias arrowtooth flounder 324-73
#> 433 4 Gadus macrocephalus Pacific cod 326-76
#> 434 3 Hippoglossus stenolepis Pacific halibut 324-73
#> 435 4 Lepidopsetta polyxystra northern rock sole 326-76
#> 436 5 Gadus macrocephalus Pacific cod 320-73
#> 437 7 Porifera sponge unid. 325-76
#> 438 3 Lepidopsetta polyxystra northern rock sole 326-76
#> 439 3 Hippoglossoides elassodon flathead sole 324-73
#> 440 3 Chionoecetes bairdi Tanner crab 324-73
#> 441 3 Hemilepidotus jordani yellow Irish lord 326-76
#> 442 4 Hippoglossus stenolepis Pacific halibut 326-76
#> 443 7 Hemilepidotus jordani yellow Irish lord 325-76
#> 444 7 Lepidopsetta bilineata southern rock sole 325-76
#> 445 3 Hippoglossoides elassodon flathead sole 326-76
#> 446 3 Gadus macrocephalus Pacific cod 324-73
#> 447 4 Gadus chalcogrammus walleye pollock 326-76
#> 448 7 Atheresthes stomias arrowtooth flounder 325-76
#> 449 3 Glyptocephalus zachirus rex sole 324-73
#> 450 5 Atheresthes stomias arrowtooth flounder 320-73
#> 451 3 Hippoglossus stenolepis Pacific halibut 326-76
#> 452 4 Atheresthes stomias arrowtooth flounder 326-76
#> 453 4 Lepidopsetta bilineata southern rock sole 326-76
#> 454 4 Hippoglossoides elassodon flathead sole 326-76
#> 455 3 Enteroctopus dofleini giant octopus 324-73
#> 456 3 Hemilepidotus jordani yellow Irish lord 324-73
#> 457 3 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 458 4 Hemilepidotus jordani yellow Irish lord 326-76
#> 459 3 Arctoraja parmifera Alaska skate 324-73
#> 460 7 Hexagrammos decagrammus kelp greenling 325-76
#> 461 3 Gymnocanthus galeatus armorhead sculpin 324-73
#> 462 3 Lepidopsetta polyxystra northern rock sole 324-73
#> 463 3 Sebastes variabilis dusky rockfish 324-73
#> 464 3 Porifera sponge unid. 324-73
#> 465 7 Sebastes alutus Pacific ocean perch 325-76
#> 466 3 Atheresthes evermanni Kamchatka flounder 326-76
#> 467 4 Gymnocanthus galeatus armorhead sculpin 326-76
#> 468 3 Sebastes alutus Pacific ocean perch 324-73
#> 469 3 Atheresthes evermanni Kamchatka flounder 324-73
#> 470 3 Podothecus accipenserinus sturgeon poacher 324-73
#> 471 7 Gymnocanthus galeatus armorhead sculpin 325-76
#> 472 3 Polychaeta polychaete worm unid. 324-73
#> 473 3 Triglops forficatus scissortail sculpin 326-76
#> 474 4 Porifera sponge unid. 326-76
#> 475 3 Ascidiacea tunicate unid. 326-76
#> 476 3 Pagurus brandti sponge hermit 324-73
#> 477 3 Hemitripterus bolini bigmouth sculpin 326-76
#> 478 3 Lepidopsetta bilineata southern rock sole 326-76
#> 479 3 Pagurus aleuticus Aleutian hermit 324-73
#> 480 3 Sebastes polyspinis northern rockfish 324-73
#> 481 5 Pennatuloidea sea whip or sea pen unid. 320-73
#> 482 4 Actiniaria sea anemone unid. 326-76
#> 483 3 Porifera sponge unid. 326-76
#> 484 4 Elassochirus tenuimanus widehand hermit crab 326-76
#> 485 3 Diplopteraster multipes pincushion sea star 326-76
#> 486 4 Holothuroidea sea cucumber unid. 326-76
#> 487 3 Ceramaster patagonicus orange bat sea star 326-76
#> 488 3 Actiniaria sea anemone unid. 326-76
#> 489 4 Pagurus ochotensis Alaskan hermit 326-76
#> 490 3 Hyas lyratus Pacific lyre crab 324-73
#> 491 5 Pagurus brandti sponge hermit 320-73
#> 492 5 Hirudinea leech unid. 320-73
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 417 2081 2536.790 1.088185e+05 89266.85531
#> 418 1164 2038.610 8.903763e+04 50838.46562
#> 419 442 651.820 2.843245e+04 19280.08511
#> 420 355 390.730 1.704368e+04 15485.13623
#> 421 142 223.770 9.541060e+03 6054.56700
#> 422 342 206.376 9.082907e+03 15051.91591
#> 423 45 148.840 6.384661e+03 1930.32604
#> 424 175 102.930 4.530099e+03 7702.00375
#> 425 160 76.840 3.296139e+03 6863.38148
#> 426 71 70.647 3.030483e+03 3045.62553
#> 427 44 69.570 3.034651e+03 1919.28449
#> 428 97 56.720 2.474132e+03 4231.14990
#> 429 70 48.200 2.067594e+03 3002.72939
#> 430 29 45.360 1.945769e+03 1243.98789
#> 431 56 45.138 1.986589e+03 2464.64120
#> 432 195 44.886 1.913840e+03 8314.37017
#> 433 14 44.050 1.923913e+03 611.45921
#> 434 26 43.582 1.858240e+03 1108.58269
#> 435 76 42.580 1.859710e+03 3319.34999
#> 436 22 40.480 1.781583e+03 968.25190
#> 437 NA 40.012 1.716360e+03 0.00000
#> 438 71 32.540 1.419398e+03 3097.02725
#> 439 173 31.884 1.359463e+03 7376.33867
#> 440 54 31.000 1.321772e+03 2302.44097
#> 441 45 30.980 1.351351e+03 1962.90459
#> 442 42 28.413 1.240956e+03 1834.37763
#> 443 31 27.840 1.194228e+03 1329.78016
#> 444 33 26.020 1.116157e+03 1415.57243
#> 445 45 25.640 1.118419e+03 1962.90459
#> 446 11 21.150 9.017894e+02 469.01575
#> 447 17 20.290 8.861791e+02 742.48618
#> 448 21 12.960 5.559339e+02 900.81882
#> 449 39 12.690 5.410736e+02 1662.87404
#> 450 7 12.440 5.475024e+02 308.08015
#> 451 9 11.320 4.937796e+02 392.58092
#> 452 19 10.420 4.551004e+02 829.83750
#> 453 10 8.040 3.511523e+02 436.75658
#> 454 8 6.260 2.734096e+02 349.40526
#> 455 1 4.060 1.731095e+02 42.63780
#> 456 6 3.952 1.685046e+02 255.82677
#> 457 2 3.930 1.675665e+02 85.27559
#> 458 5 3.920 1.712086e+02 218.37829
#> 459 1 3.560 1.517906e+02 42.63780
#> 460 5 3.400 1.458469e+02 214.48067
#> 461 13 3.324 1.417280e+02 554.29134
#> 462 2 1.250 5.329724e+01 85.27559
#> 463 3 0.974 4.152921e+01 127.91339
#> 464 NA 0.942 4.016480e+01 0.00000
#> 465 9 0.936 4.015078e+01 386.06521
#> 466 1 0.722 3.149371e+01 43.62010
#> 467 3 0.700 3.057296e+01 131.02697
#> 468 8 0.678 2.890843e+01 341.10237
#> 469 6 0.642 2.737346e+01 255.82677
#> 470 7 0.550 2.345079e+01 298.46457
#> 471 2 0.484 2.076173e+01 85.79227
#> 472 NA 0.272 1.159748e+01 0.00000
#> 473 3 0.260 1.134123e+01 130.86031
#> 474 NA 0.258 1.126832e+01 0.00000
#> 475 1 0.216 9.421942e+00 43.62010
#> 476 8 0.168 7.163150e+00 341.10237
#> 477 1 0.168 7.328177e+00 43.62010
#> 478 1 0.150 6.543015e+00 43.62010
#> 479 2 0.134 5.713465e+00 85.27559
#> 480 1 0.106 4.519606e+00 42.63780
#> 481 NA 0.090 3.961031e+00 0.00000
#> 482 2 0.088 3.843458e+00 87.35132
#> 483 NA 0.070 3.053407e+00 0.00000
#> 484 2 0.066 2.882593e+00 87.35132
#> 485 1 0.062 2.704446e+00 43.62010
#> 486 1 0.052 2.271134e+00 43.67566
#> 487 1 0.026 1.134123e+00 43.62010
#> 488 1 0.022 9.596420e-01 43.62010
#> 489 1 0.016 6.988110e-01 43.67566
#> 490 1 0.010 4.263780e-01 42.63780
#> 491 1 0.008 3.520920e-01 44.01145
#> 492 1 0.004 1.760460e-01 44.01145
#>
#> $catch$`2012`
#> haul scientific_name common_name station count
#> 318 6 Lepidopsetta bilineata southern rock sole 321-74 310
#> 319 6 Gadus chalcogrammus walleye pollock 321-74 60
#> 320 4 Gadus chalcogrammus walleye pollock 326-76 76
#> 321 6 Hippoglossus stenolepis Pacific halibut 321-74 80
#> 322 7 Lepidopsetta polyxystra northern rock sole 320-73 82
#> 323 6 Lepidopsetta polyxystra northern rock sole 321-74 51
#> 324 1 Gadus macrocephalus Pacific cod 326-76 17
#> 325 7 Lepidopsetta bilineata southern rock sole 320-73 49
#> 326 6 Gadus macrocephalus Pacific cod 321-74 16
#> 327 1 Hippoglossus stenolepis Pacific halibut 320-74 25
#> 328 7 Hippoglossus stenolepis Pacific halibut 320-74 15
#> 329 4 Lepidopsetta polyxystra northern rock sole 326-76 29
#> 330 6 Atheresthes stomias arrowtooth flounder 321-74 15
#> 331 7 Hippoglossus stenolepis Pacific halibut 320-73 18
#> 332 1 Lepidopsetta polyxystra northern rock sole 326-76 26
#> 333 1 Lepidopsetta polyxystra northern rock sole 320-74 17
#> 334 1 Hemilepidotus jordani yellow Irish lord 326-76 18
#> 335 1 Atheresthes stomias arrowtooth flounder 326-76 15
#> 336 4 Hippoglossus stenolepis Pacific halibut 326-76 11
#> 337 1 Gadus macrocephalus Pacific cod 320-74 6
#> 338 4 Atheresthes stomias arrowtooth flounder 326-76 15
#> 339 7 Atheresthes stomias arrowtooth flounder 320-74 8
#> 340 1 Atheresthes stomias arrowtooth flounder 320-74 20
#> 341 7 Atheresthes stomias arrowtooth flounder 320-73 7
#> 342 4 Gadus macrocephalus Pacific cod 326-76 4
#> 343 6 Hippoglossoides elassodon flathead sole 321-74 7
#> 344 7 Glyptocephalus zachirus rex sole 320-74 6
#> 345 4 Hippoglossoides elassodon flathead sole 326-76 8
#> 346 1 Gadus chalcogrammus walleye pollock 320-74 2
#> 347 7 Gadus macrocephalus Pacific cod 320-73 4
#> 348 1 Lepidopsetta bilineata southern rock sole 320-74 4
#> 349 7 Pleurogrammus monopterygius Atka mackerel 320-73 2
#> 350 7 Hemilepidotus jordani yellow Irish lord 320-74 3
#> 351 4 Pleurogrammus monopterygius Atka mackerel 326-76 2
#> 352 7 Gadus chalcogrammus walleye pollock 320-74 2
#> 353 1 Glyptocephalus zachirus rex sole 320-74 4
#> 354 1 Pleurogrammus monopterygius Atka mackerel 326-76 2
#> 355 7 Lepidopsetta polyxystra northern rock sole 320-74 2
#> 356 1 Gadus chalcogrammus walleye pollock 326-76 1
#> 357 1 Porifera sponge unid. 326-76 NA
#> 358 7 Gadus macrocephalus Pacific cod 320-74 1
#> 359 4 Hemilepidotus jordani yellow Irish lord 326-76 2
#> 360 6 Berryteuthis magister magistrate armhook squid 321-74 5
#> 361 1 Hippoglossus stenolepis Pacific halibut 326-76 2
#> 362 7 Hemilepidotus jordani yellow Irish lord 320-73 1
#> 363 1 Hemilepidotus jordani yellow Irish lord 320-74 2
#> 364 1 Ascidiacea tunicate unid. 326-76 21
#> 365 6 Hemilepidotus jordani yellow Irish lord 321-74 1
#> 366 7 Hippoglossoides elassodon flathead sole 320-74 2
#> 367 1 Hippoglossoides elassodon flathead sole 326-76 2
#> 368 7 Berryteuthis magister magistrate armhook squid 320-74 3
#> 369 4 Lepidopsetta bilineata southern rock sole 326-76 1
#> 370 7 Atheresthes evermanni Kamchatka flounder 320-73 2
#> 371 1 Berryteuthis magister magistrate armhook squid 326-76 2
#> 372 7 Hemilepidotus hemilepidotus red Irish lord 320-73 1
#> 373 1 Hippoglossoides elassodon flathead sole 320-74 1
#> 374 4 Gymnocanthus galeatus armorhead sculpin 326-76 1
#> 375 1 Berryteuthis magister magistrate armhook squid 320-74 1
#> 376 4 Berryteuthis magister magistrate armhook squid 326-76 1
#> 377 1 Atheresthes evermanni Kamchatka flounder 326-76 1
#> 378 7 Ascidiacea tunicate unid. 320-73 1
#> 379 4 Gorgonocephalus eucnemis basketstar 326-76 1
#> 380 4 Triglops forficatus scissortail sculpin 326-76 3
#> 381 4 Atheresthes evermanni Kamchatka flounder 326-76 2
#> 382 1 Modiolus modiolus northern horsemussel 326-76 4
#> 383 1 Bathymaster signatus searcher 326-76 1
#> 384 1 Actiniaria sea anemone unid. 326-76 2
#> 385 6 Gorgonocephalus eucnemis basketstar 321-74 1
#> 386 1 Elassochirus cavimanus purple hermit 326-76 3
#> 387 1 Actiniaria sea anemone unid. 320-74 2
#> 388 7 Gorgonocephalus eucnemis basketstar 320-74 1
#> 389 1 Sebastes alutus Pacific ocean perch 326-76 2
#> 390 1 Bryozoa bryozoan unid. 326-76 NA
#> 391 4 Thaliacea salp unid. 326-76 1
#> 392 7 Triglops scepticus spectacled sculpin 320-74 2
#> 393 1 Pagurus ochotensis Alaskan hermit 326-76 3
#> 394 1 Pagurus confragosus knobbyhand hermit 326-76 1
#> 395 1 Triglops forficatus scissortail sculpin 326-76 1
#> 396 1 Pennatuloidea sea whip or sea pen unid. 326-76 1
#> 397 1 Scyphozoa jellyfish unid. 320-74 1
#> 398 1 Sarritor frenatus sawback poacher 326-76 1
#> 399 1 Triglops scepticus spectacled sculpin 326-76 1
#> 400 1 Lethasterias nanimensis blackspined sea star 326-76 1
#> 401 6 Cheiraster dawsoni fragile sea star 321-74 1
#> 402 1 Hyas lyratus Pacific lyre crab 326-76 3
#> 403 7 Gadus chalcogrammus walleye pollock 320-73 1
#> 404 7 Porifera sponge unid. 320-73 NA
#> 405 1 Pagurus aleuticus Aleutian hermit 326-76 1
#> 406 1 Nudibranchia nudibranch unid. 326-76 1
#> 407 7 Ascidiacea tunicate unid. 320-74 3
#> 408 6 Pagurus aleuticus Aleutian hermit 321-74 1
#> 409 1 Ophiuroidea brittlestar unid. 326-76 3
#> 410 1 Cheiraster dawsoni fragile sea star 326-76 1
#> 411 1 Brachiopoda lampshell unid. 326-76 1
#> 412 1 Eumicrotremus sp. spiny lumpsuckers 326-76 2
#> 413 1 Ophiuroidea brittlestar unid. 320-74 1
#> 414 1 Porifera sponge unid. 320-74 NA
#> 415 7 Bulbus fragilis fragile moonsnail 320-73 1
#> 416 1 Isopoda isopod unid. 326-76 1
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 318 266.120 11732.310055 13666.82744
#> 319 99.810 4400.277569 2645.19241
#> 320 68.020 3199.438570 3574.79170
#> 321 57.449 2532.727643 3526.92321
#> 322 47.540 2076.651323 3581.93960
#> 323 34.780 1533.329865 2248.41355
#> 324 29.110 1295.747314 756.70575
#> 325 28.570 1248.000175 2140.42732
#> 326 20.500 903.774072 705.38464
#> 327 20.490 947.837132 1156.46307
#> 328 17.956 795.845365 664.82961
#> 329 12.690 596.896140 1364.06525
#> 330 11.450 504.790884 661.29810
#> 331 11.422 498.937977 786.27942
#> 332 10.610 472.273411 1157.31467
#> 333 9.930 459.347131 786.39489
#> 334 9.560 425.535703 801.21785
#> 335 9.300 413.962556 667.68154
#> 336 8.163 383.960850 517.40406
#> 337 7.590 351.102188 277.55114
#> 338 6.380 300.094356 705.55099
#> 339 6.040 267.704723 354.57579
#> 340 4.780 221.115739 925.17046
#> 341 4.700 205.306294 305.77533
#> 342 4.620 217.309706 188.14693
#> 343 4.430 195.303373 308.60578
#> 344 3.900 172.855698 265.93184
#> 345 3.670 172.624810 376.29386
#> 346 3.460 160.054489 92.51705
#> 347 3.450 150.703556 174.72876
#> 348 3.250 150.340199 185.03409
#> 349 2.930 127.988817 87.36438
#> 350 2.496 110.627647 132.96592
#> 351 2.210 103.951180 94.07347
#> 352 1.946 86.250561 88.64395
#> 353 1.772 81.970102 185.03409
#> 354 1.710 76.115696 89.02421
#> 355 1.608 71.269734 88.64395
#> 356 1.540 68.548638 44.51210
#> 357 1.505 66.990715 0.00000
#> 358 1.450 64.266862 44.32197
#> 359 1.400 65.851426 94.07347
#> 360 1.372 60.486733 220.43270
#> 361 1.107 49.274898 89.02421
#> 362 0.962 42.022267 43.68219
#> 363 0.926 42.835392 92.51705
#> 364 0.916 40.773086 934.75416
#> 365 0.774 34.122982 44.08654
#> 366 0.745 33.019871 88.64395
#> 367 0.640 28.487746 89.02421
#> 368 0.604 26.770472 132.96592
#> 369 0.604 28.410187 47.03673
#> 370 0.515 22.496328 87.36438
#> 371 0.495 22.033491 89.02421
#> 372 0.390 17.036054 43.68219
#> 373 0.328 15.172795 46.25852
#> 374 0.312 14.675461 47.03673
#> 375 0.274 12.674835 46.25852
#> 376 0.262 12.323624 47.03673
#> 377 0.234 10.415832 44.51210
#> 378 0.222 9.697446 43.68219
#> 379 0.210 9.877714 47.03673
#> 380 0.196 9.219200 141.11020
#> 381 0.180 8.466612 94.07347
#> 382 0.180 8.012179 178.04841
#> 383 0.132 5.875598 44.51210
#> 384 0.120 5.341452 89.02421
#> 385 0.110 4.849519 44.08654
#> 386 0.100 4.451210 133.53631
#> 387 0.084 3.885716 92.51705
#> 388 0.078 3.457114 44.32197
#> 389 0.074 3.293896 89.02421
#> 390 0.070 3.115847 0.00000
#> 391 0.070 3.292571 47.03673
#> 392 0.056 2.482031 88.64395
#> 393 0.055 2.448166 133.53631
#> 394 0.055 2.448166 44.51210
#> 395 0.052 2.314629 44.51210
#> 396 0.050 2.225605 44.51210
#> 397 0.048 2.220409 46.25852
#> 398 0.048 2.136581 44.51210
#> 399 0.032 1.424387 44.51210
#> 400 0.030 1.335363 44.51210
#> 401 0.028 1.234423 44.08654
#> 402 0.025 1.112803 133.53631
#> 403 0.024 1.048373 43.68219
#> 404 0.020 0.873644 0.00000
#> 405 0.015 0.667682 44.51210
#> 406 0.015 0.667682 44.51210
#> 407 0.014 0.620508 132.96592
#> 408 0.012 0.529038 44.08654
#> 409 0.010 0.445121 133.53631
#> 410 0.010 0.445121 44.51210
#> 411 0.010 0.445121 44.51210
#> 412 0.010 0.445121 89.02421
#> 413 0.008 0.370068 46.25852
#> 414 0.008 0.370068 0.00000
#> 415 0.005 0.218411 43.68219
#> 416 0.005 0.222561 44.51210
#>
#> $catch$`2014`
#> haul scientific_name common_name station count
#> 210 3 Pleurogrammus monopterygius Atka mackerel 320-74 142
#> 211 3 Atheresthes stomias arrowtooth flounder 320-74 129
#> 212 6 Lepidopsetta polyxystra northern rock sole 320-74 298
#> 213 3 Lepidopsetta polyxystra northern rock sole 320-74 172
#> 214 1 Hippoglossoides elassodon flathead sole 321-74 129
#> 215 5 Gadus macrocephalus Pacific cod 326-76 74
#> 216 1 Lepidopsetta bilineata southern rock sole 321-74 66
#> 217 1 Gadus macrocephalus Pacific cod 321-74 16
#> 218 6 Gadus macrocephalus Pacific cod 320-74 11
#> 219 6 Lepidopsetta bilineata southern rock sole 320-74 34
#> 220 3 Gadus macrocephalus Pacific cod 320-74 39
#> 221 3 Hippoglossus stenolepis Pacific halibut 320-74 11
#> 222 3 Enteroctopus dofleini giant octopus 320-74 3
#> 223 3 Gadus chalcogrammus walleye pollock 320-74 15
#> 224 3 Sebastes variabilis dusky rockfish 320-74 22
#> 225 1 Atheresthes stomias arrowtooth flounder 321-74 99
#> 226 3 Hemilepidotus jordani yellow Irish lord 320-74 18
#> 227 5 Atheresthes stomias arrowtooth flounder 326-76 35
#> 228 5 Lepidopsetta polyxystra northern rock sole 326-76 37
#> 229 6 Hippoglossus stenolepis Pacific halibut 320-74 11
#> 230 5 Hemilepidotus jordani yellow Irish lord 326-76 20
#> 231 3 Sebastes polyspinis northern rockfish 320-74 17
#> 232 5 Hippoglossus stenolepis Pacific halibut 326-76 7
#> 233 1 Enteroctopus dofleini giant octopus 321-74 1
#> 234 1 Actiniaria sea anemone unid. 321-74 39
#> 235 3 Atheresthes evermanni Kamchatka flounder 320-74 10
#> 236 3 Hippoglossoides elassodon flathead sole 320-74 10
#> 237 3 Glyptocephalus zachirus rex sole 320-74 7
#> 238 1 Hippoglossus stenolepis Pacific halibut 321-74 4
#> 239 6 Anarrhichthys ocellatus wolf-eel 320-74 1
#> 240 1 Gadus chalcogrammus walleye pollock 321-74 2
#> 241 3 Sebastes alutus Pacific ocean perch 320-74 8
#> 242 1 Porifera sponge unid. 321-74 NA
#> 243 3 Lepidopsetta bilineata southern rock sole 320-74 3
#> 244 5 Hippoglossoides elassodon flathead sole 326-76 3
#> 245 1 Lepidopsetta polyxystra northern rock sole 321-74 4
#> 246 3 Bathyraja taranetzi mud skate 320-74 1
#> 247 5 Pleurogrammus monopterygius Atka mackerel 326-76 2
#> 248 5 Hemitripterus bolini bigmouth sculpin 326-76 2
#> 249 5 Triglops forficatus scissortail sculpin 326-76 12
#> 250 6 Atheresthes stomias arrowtooth flounder 320-74 9
#> 251 6 Aptocyclus ventricosus smooth lumpsucker 320-74 1
#> 252 6 Hemilepidotus jordani yellow Irish lord 320-74 1
#> 253 5 Lepidopsetta bilineata southern rock sole 326-76 1
#> 254 5 Atheresthes evermanni Kamchatka flounder 326-76 2
#> 255 3 Sebastes melanostictus blackspotted rockfish 320-74 1
#> 256 3 Malacocottus zonurus darkfin sculpin 320-74 1
#> 257 5 Porifera sponge unid. 326-76 NA
#> 258 6 Hippoglossoides elassodon flathead sole 320-74 1
#> 259 1 Pleurogrammus monopterygius Atka mackerel 321-74 1
#> 260 1 Thaliacea salp unid. 321-74 NA
#> 261 3 Holothuroidea sea cucumber unid. 320-74 11
#> 262 3 Pagurus brandti sponge hermit 320-74 17
#> 263 3 Porifera sponge unid. 320-74 1
#> 264 5 Gadus chalcogrammus walleye pollock 326-76 2
#> 265 1 Polychaeta polychaete worm unid. 321-74 NA
#> 266 5 Modiolus modiolus northern horsemussel 326-76 1
#> 267 3 Lethasterias nanimensis blackspined sea star 320-74 1
#> 268 3 Actiniaria sea anemone unid. 320-74 3
#> 269 3 Elassochirus cavimanus purple hermit 320-74 10
#> 270 5 Sebastes alutus Pacific ocean perch 326-76 2
#> 271 3 Scyphozoa jellyfish unid. 320-74 NA
#> 272 3 Sebastes ciliatus dark rockfish 320-74 1
#> 273 6 Crystallichthys cyclospilus blotched snailfish 320-74 1
#> 274 5 Scyphozoa jellyfish unid. 326-76 1
#> 275 3 Pagurus cornutus hornyhand hermit 320-74 5
#> 276 3 Solaster dawsoni morning sun sea star 320-74 1
#> 277 6 Ophiuroidea brittlestar unid. 320-74 16
#> 278 1 Atheresthes evermanni Kamchatka flounder 321-74 4
#> 279 3 Ophiuroidea brittlestar unid. 320-74 17
#> 280 3 Pagurus confragosus knobbyhand hermit 320-74 7
#> 281 6 Porifera sponge unid. 320-74 NA
#> 282 6 Gymnocanthus galeatus armorhead sculpin 320-74 2
#> 283 3 Hyas lyratus Pacific lyre crab 320-74 7
#> 284 3 Triglops scepticus spectacled sculpin 320-74 4
#> 285 1 Ophiuroidea brittlestar unid. 321-74 25
#> 286 3 Ascidiacea tunicate unid. 320-74 1
#> 287 5 Crystallichthys cyclospilus blotched snailfish 326-76 1
#> 288 1 Podothecus accipenserinus sturgeon poacher 321-74 1
#> 289 6 Holothuroidea sea cucumber unid. 320-74 2
#> 290 5 Ceramaster japonicus red bat star 326-76 1
#> 291 5 Hyas lyratus Pacific lyre crab 326-76 1
#> 292 3 Diplopteraster multipes pincushion sea star 320-74 1
#> 293 1 Elassochirus cavimanus purple hermit 321-74 1
#> 294 6 Actiniaria sea anemone unid. 320-74 3
#> 295 5 Elassochirus cavimanus purple hermit 326-76 1
#> 296 1 Hyas lyratus Pacific lyre crab 321-74 1
#> 297 5 Nudibranchia nudibranch unid. 326-76 1
#> 298 5 Pseudarchaster parelii scarlet sea star 326-76 1
#> 299 6 Elassochirus tenuimanus widehand hermit crab 320-74 2
#> 300 1 Pagurus brandti sponge hermit 321-74 2
#> 301 3 Cheiraster dawsoni fragile sea star 320-74 2
#> 302 3 Castaneobuccinum castaneum chestnut whelk 320-74 1
#> 303 6 Polychaeta polychaete worm unid. 320-74 1
#> 304 5 Aptocyclus ventricosus smooth lumpsucker 326-76 3
#> 305 1 Modiolus modiolus northern horsemussel 321-74 1
#> 306 6 Ceramaster arcticus Arctic bat sea star 320-74 1
#> 307 6 Hyas lyratus Pacific lyre crab 320-74 1
#> 308 5 Pedicellaster magister majestic sea star 326-76 1
#> 309 3 Keenocardium blandum low-rib cockle 320-74 1
#> 310 3 Polychaeta polychaete worm unid. 320-74 2
#> 311 3 Labidochirus splendescens splendid hermit 320-74 1
#> 312 1 Scyphozoa jellyfish unid. 321-74 1
#> 313 1 Rhamphocottus richardsonii grunt sculpin 321-74 1
#> 314 6 Bryozoa bryozoan unid. 320-74 NA
#> 315 3 Paguridae hermit crab unid. 320-74 3
#> 316 1 Bryozoa bryozoan unid. 321-74 NA
#> 317 5 Hydroidolina hydroid unid. 326-76 NA
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 210 214.240 8637.949677 5725.30272
#> 211 129.014 5201.719752 5201.15528
#> 212 126.552 5725.758380 13482.80546
#> 213 110.220 4443.963842 6934.87371
#> 214 97.520 4081.346355 5398.82773
#> 215 67.910 3286.122879 3580.81421
#> 216 62.090 2598.552043 2762.19093
#> 217 38.570 1614.207639 669.62204
#> 218 36.530 1652.774777 497.68745
#> 219 32.038 1449.537320 1538.30666
#> 220 28.920 1166.026441 1572.44230
#> 221 28.484 1148.447342 443.50937
#> 222 26.480 1067.648000 120.95710
#> 223 19.220 774.931818 604.78550
#> 224 17.860 720.097933 887.01873
#> 225 15.930 666.692447 4143.28640
#> 226 13.590 547.935661 725.74260
#> 227 13.480 652.288859 1693.62834
#> 228 11.940 577.769212 1790.40711
#> 229 11.383 515.016022 497.68745
#> 230 11.180 540.993282 967.78762
#> 231 11.150 449.557220 685.42357
#> 232 10.763 520.814910 338.72567
#> 233 10.250 428.976622 41.85138
#> 234 9.998 418.430074 1632.20373
#> 235 6.080 245.139722 403.19033
#> 236 5.780 233.044012 403.19033
#> 237 5.670 228.608918 282.23323
#> 238 5.228 218.799003 167.40551
#> 239 4.480 202.694525 45.24431
#> 240 4.020 168.242538 83.70275
#> 241 3.680 148.374042 322.55227
#> 242 3.276 137.105113 0.00000
#> 243 2.790 112.490103 120.95710
#> 244 2.260 109.360002 145.16814
#> 245 2.008 84.037566 167.40551
#> 246 1.722 69.429375 40.31903
#> 247 1.680 81.294160 96.77876
#> 248 1.620 78.390798 96.77876
#> 249 1.288 62.325523 580.67258
#> 250 1.216 55.017085 407.19882
#> 251 1.110 50.221188 45.24431
#> 252 0.992 44.882359 45.24431
#> 253 0.920 44.518231 48.38938
#> 254 0.840 40.647080 96.77876
#> 255 0.770 31.045656 40.31903
#> 256 0.732 29.513532 40.31903
#> 257 0.632 30.582089 0.00000
#> 258 0.618 27.960986 45.24431
#> 259 0.580 24.273799 41.85138
#> 260 0.564 23.604177 0.00000
#> 261 0.440 17.740375 443.50937
#> 262 0.364 14.676128 685.42357
#> 263 0.348 14.031024 40.31903
#> 264 0.304 14.710372 96.77876
#> 265 0.296 12.388008 0.00000
#> 266 0.284 13.742584 48.38938
#> 267 0.274 11.047415 40.31903
#> 268 0.270 10.886139 120.95710
#> 269 0.240 9.676568 403.19033
#> 270 0.236 11.419894 96.77876
#> 271 0.200 8.063807 0.00000
#> 272 0.198 7.983169 40.31903
#> 273 0.178 8.053488 45.24431
#> 274 0.168 8.129416 48.38938
#> 275 0.156 6.289769 201.59517
#> 276 0.136 5.483389 40.31903
#> 277 0.132 5.972249 723.90902
#> 278 0.126 5.273274 167.40551
#> 279 0.116 4.677008 685.42357
#> 280 0.110 4.435094 282.23323
#> 281 0.106 4.795897 0.00000
#> 282 0.104 4.705409 90.48863
#> 283 0.100 4.031903 282.23323
#> 284 0.098 3.951265 161.27613
#> 285 0.098 4.101435 1046.28444
#> 286 0.088 3.548075 40.31903
#> 287 0.084 4.064708 48.38938
#> 288 0.076 3.180705 41.85138
#> 289 0.060 2.714659 90.48863
#> 290 0.060 2.903363 48.38938
#> 291 0.058 2.806584 48.38938
#> 292 0.054 2.177228 40.31903
#> 293 0.050 2.092569 41.85138
#> 294 0.048 2.171727 135.73294
#> 295 0.042 2.032354 48.38938
#> 296 0.036 1.506650 41.85138
#> 297 0.036 1.742018 48.38938
#> 298 0.034 1.645239 48.38938
#> 299 0.032 1.447818 90.48863
#> 300 0.030 1.255541 83.70275
#> 301 0.022 0.887019 80.63807
#> 302 0.018 0.725743 40.31903
#> 303 0.018 0.814398 45.24431
#> 304 0.018 0.871009 145.16814
#> 305 0.016 0.669622 41.85138
#> 306 0.014 0.633420 45.24431
#> 307 0.010 0.452443 45.24431
#> 308 0.010 0.483894 48.38938
#> 309 0.008 0.322552 40.31903
#> 310 0.008 0.322552 80.63807
#> 311 0.006 0.241914 40.31903
#> 312 0.006 0.251108 41.85138
#> 313 0.004 0.167406 41.85138
#> 314 0.002 0.090489 0.00000
#> 315 0.002 0.080638 120.95710
#> 316 0.002 0.083703 0.00000
#> 317 0.002 0.096779 0.00000
#>
#> $catch$`2016`
#> haul scientific_name common_name station
#> 135 3 Gadus macrocephalus Pacific cod 320-73
#> 136 7 Atheresthes stomias arrowtooth flounder 320-74
#> 137 3 Hippoglossus stenolepis Pacific halibut 320-73
#> 138 5 Hippoglossoides elassodon flathead sole 320-74
#> 139 5 Lepidopsetta bilineata southern rock sole 320-74
#> 140 3 Lepidopsetta bilineata southern rock sole 320-73
#> 141 3 Lepidopsetta polyxystra northern rock sole 320-73
#> 142 5 Hippoglossus stenolepis Pacific halibut 320-74
#> 143 4 Hippoglossoides elassodon flathead sole 324-73
#> 144 7 Gadus macrocephalus Pacific cod 320-74
#> 145 4 Atheresthes stomias arrowtooth flounder 324-73
#> 146 7 Lepidopsetta polyxystra northern rock sole 320-74
#> 147 3 Atheresthes stomias arrowtooth flounder 320-73
#> 148 7 Hippoglossus stenolepis Pacific halibut 320-74
#> 149 4 Hippoglossus stenolepis Pacific halibut 324-73
#> 150 7 Enteroctopus dofleini giant octopus 320-74
#> 151 5 Lepidopsetta polyxystra northern rock sole 320-74
#> 152 4 Anoplopoma fimbria sablefish 324-73
#> 153 4 Gadus chalcogrammus walleye pollock 324-73
#> 154 4 Lepidopsetta bilineata southern rock sole 324-73
#> 155 5 Atheresthes stomias arrowtooth flounder 320-74
#> 156 4 Parophrys vetulus English sole 324-73
#> 157 4 Glyptocephalus zachirus rex sole 324-73
#> 158 5 Gadus macrocephalus Pacific cod 320-74
#> 159 3 Anarrhichthys ocellatus wolf-eel 320-73
#> 160 4 Enteroctopus dofleini giant octopus 324-73
#> 161 4 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 162 3 Clupea pallasii Pacific herring 320-73
#> 163 7 Hemilepidotus jordani yellow Irish lord 320-74
#> 164 3 Pleurogrammus monopterygius Atka mackerel 320-73
#> 165 7 Glyptocephalus zachirus rex sole 320-74
#> 166 5 Hemilepidotus jordani yellow Irish lord 320-74
#> 167 7 Lepidopsetta bilineata southern rock sole 320-74
#> 168 4 Gadus macrocephalus Pacific cod 324-73
#> 169 7 Atheresthes evermanni Kamchatka flounder 320-74
#> 170 3 Hemilepidotus jordani yellow Irish lord 320-73
#> 171 5 Pycnopodia helianthoides sunflower sea star 320-74
#> 172 4 Gorgonocephalus eucnemis basketstar 324-73
#> 173 4 Thaliacea salp unid. 324-73
#> 174 3 Hippoglossoides elassodon flathead sole 320-73
#> 175 5 Pleurogrammus monopterygius Atka mackerel 320-74
#> 176 7 Sebastes polyspinis northern rockfish 320-74
#> 177 5 Gadus chalcogrammus walleye pollock 320-74
#> 178 7 Hippoglossoides elassodon flathead sole 320-74
#> 179 4 Atheresthes evermanni Kamchatka flounder 324-73
#> 180 4 Podothecus accipenserinus sturgeon poacher 324-73
#> 181 3 Pennatuloidea sea whip or sea pen unid. 320-73
#> 182 5 Gorgonocephalus eucnemis basketstar 320-74
#> 183 4 Sebastes polyspinis northern rockfish 324-73
#> 184 7 Pagurus trigonocheirus fuzzy hermit crab 320-74
#> 185 7 Thaliacea salp unid. 320-74
#> 186 4 Bathymaster signatus searcher 324-73
#> 187 7 Elassochirus cavimanus purple hermit 320-74
#> 188 4 Pagurus confragosus knobbyhand hermit 324-73
#> 189 7 Modiolus modiolus northern horsemussel 320-74
#> 190 5 Thaliacea salp unid. 320-74
#> 191 3 Ammodytes sp. sand lance unid. 320-73
#> 192 4 Pagurus aleuticus Aleutian hermit 324-73
#> 193 7 Pagurus confragosus knobbyhand hermit 320-74
#> 194 4 Porifera sponge unid. 324-73
#> 195 7 Ceramaster patagonicus orange bat sea star 320-74
#> 196 3 Gorgonocephalus eucnemis basketstar 320-73
#> 197 4 Polychaeta polychaete worm unid. 324-73
#> 198 5 Rajiformes egg case skate egg case unid. 320-74
#> 199 7 Bryozoa bryozoan unid. 320-74
#> 200 4 Nudibranchia nudibranch unid. 324-73
#> 201 5 Ophiuroidea brittlestar unid. 320-74
#> 202 3 Ophiuroidea brittlestar unid. 320-73
#> 203 7 Actiniaria sea anemone unid. 320-74
#> 204 4 Rajiformes egg case skate egg case unid. 324-73
#> 205 7 Hyas lyratus Pacific lyre crab 320-74
#> 206 4 Actiniaria sea anemone unid. 324-73
#> 207 4 Labidochirus splendescens splendid hermit 324-73
#> 208 7 Rajiformes egg case skate egg case unid. 320-74
#> 209 7 Ascidiacea tunicate unid. 320-74
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 135 243 343.340 14793.441181 10470.10604
#> 136 291 136.844 6214.554539 13215.30627
#> 137 133 118.374 5100.363507 5730.55186
#> 138 78 67.768 3050.003623 3510.51060
#> 139 69 62.926 2832.081926 3105.45169
#> 140 90 59.940 2582.626156 3877.81705
#> 141 103 33.600 1447.718366 4437.94618
#> 142 31 28.166 1267.654380 1395.20293
#> 143 133 23.320 950.181635 5419.13196
#> 144 10 20.740 941.874405 454.13424
#> 145 54 20.212 823.545077 2200.24907
#> 146 41 20.080 911.901546 1861.95037
#> 147 27 17.950 773.409067 1163.34512
#> 148 12 15.040 683.017891 544.96108
#> 149 11 10.780 439.234907 448.19888
#> 150 2 10.220 464.125189 90.82685
#> 151 26 9.904 445.744834 1170.17020
#> 152 11 8.980 365.893271 448.19888
#> 153 10 8.680 353.669665 407.45353
#> 154 6 8.620 351.224944 244.47212
#> 155 8 8.320 374.454464 360.05237
#> 156 6 7.000 285.217472 244.47212
#> 157 29 6.612 269.408275 1181.61524
#> 158 4 6.080 273.639801 180.02618
#> 159 1 5.900 254.212451 43.08686
#> 160 1 5.562 226.625654 40.74535
#> 161 1 4.960 202.096951 40.74535
#> 162 10 4.320 186.135218 430.86856
#> 163 6 3.920 178.020621 272.48054
#> 164 5 3.870 166.746133 215.43428
#> 165 7 3.340 151.680835 317.89396
#> 166 2 2.540 114.316627 90.01309
#> 167 2 2.468 112.080329 90.82685
#> 168 2 2.240 91.269591 81.49071
#> 169 8 1.672 75.931244 363.30739
#> 170 1 1.332 57.391692 43.08686
#> 171 1 1.320 59.408641 45.00655
#> 172 4 1.182 48.161007 162.98141
#> 173 NA 1.072 43.679019 0.00000
#> 174 1 0.946 40.760166 43.08686
#> 175 1 0.910 40.955957 45.00655
#> 176 2 0.863 39.191785 90.82685
#> 177 1 0.754 33.934936 45.00655
#> 178 1 0.648 29.427898 45.41342
#> 179 5 0.416 16.950067 203.72677
#> 180 4 0.360 14.668327 162.98141
#> 181 NA 0.258 11.116409 0.00000
#> 182 1 0.236 10.621545 45.00655
#> 183 1 0.198 8.067580 40.74535
#> 184 5 0.186 8.446897 227.06712
#> 185 NA 0.172 7.811109 0.00000
#> 186 1 0.152 6.193294 40.74535
#> 187 5 0.148 6.721187 227.06712
#> 188 5 0.142 5.785840 203.72677
#> 189 1 0.090 4.087208 45.41342
#> 190 NA 0.088 3.960576 0.00000
#> 191 2 0.080 3.446948 86.17371
#> 192 3 0.066 2.689193 122.23606
#> 193 2 0.060 2.724805 90.82685
#> 194 NA 0.060 2.444721 0.00000
#> 195 1 0.058 2.633979 45.41342
#> 196 1 0.056 2.412864 43.08686
#> 197 NA 0.054 2.200249 0.00000
#> 198 4 0.048 2.160314 180.02618
#> 199 NA 0.044 1.998191 0.00000
#> 200 1 0.036 1.466833 40.74535
#> 201 6 0.032 1.440209 270.03928
#> 202 5 0.020 0.861737 215.43428
#> 203 1 0.020 0.908268 45.41342
#> 204 NA 0.020 0.814907 0.00000
#> 205 2 0.018 0.817442 90.82685
#> 206 1 0.016 0.651926 40.74535
#> 207 1 0.008 0.325963 40.74535
#> 208 NA 0.004 0.181654 0.00000
#> 209 NA 0.002 0.090827 0.00000
#>
#> $catch$`2018`
#> haul scientific_name common_name station
#> 54 2 Gadus chalcogrammus walleye pollock 325-76
#> 55 9 Atheresthes stomias arrowtooth flounder 320-74
#> 56 1 Gadus chalcogrammus walleye pollock 324-73
#> 57 2 Porifera sponge unid. 325-76
#> 58 1 Atheresthes stomias arrowtooth flounder 324-73
#> 59 9 Lepidopsetta polyxystra northern rock sole 320-74
#> 60 2 Sebastes polyspinis northern rockfish 325-76
#> 61 2 Gadus macrocephalus Pacific cod 325-76
#> 62 9 Gadus chalcogrammus walleye pollock 320-74
#> 63 9 Gadus macrocephalus Pacific cod 320-74
#> 64 2 Atheresthes stomias arrowtooth flounder 325-76
#> 65 9 Hemilepidotus jordani yellow Irish lord 320-74
#> 66 9 Hippoglossus stenolepis Pacific halibut 320-74
#> 67 9 Bathyraja aleutica Aleutian skate 320-74
#> 68 1 Hippoglossus stenolepis Pacific halibut 324-73
#> 69 9 Sebastes alutus Pacific ocean perch 320-74
#> 70 1 Gadus macrocephalus Pacific cod 324-73
#> 71 2 Lepidopsetta polyxystra northern rock sole 325-76
#> 72 10 Gadus macrocephalus Pacific cod 320-73
#> 73 2 Enteroctopus dofleini giant octopus 325-76
#> 74 10 Hippoglossus stenolepis Pacific halibut 320-73
#> 75 2 Hemilepidotus jordani yellow Irish lord 325-76
#> 76 2 Hexagrammos decagrammus kelp greenling 325-76
#> 77 1 Hippoglossoides elassodon flathead sole 324-73
#> 78 2 Hippoglossus stenolepis Pacific halibut 325-76
#> 79 9 Sebastes polyspinis northern rockfish 320-74
#> 80 9 Glyptocephalus zachirus rex sole 320-74
#> 81 9 Lepidopsetta bilineata southern rock sole 320-74
#> 82 1 Chionoecetes bairdi Tanner crab 324-73
#> 83 1 Glyptocephalus zachirus rex sole 324-73
#> 84 2 Pleurogrammus monopterygius Atka mackerel 325-76
#> 85 9 Enteroctopus dofleini giant octopus 320-74
#> 86 9 Hippoglossoides elassodon flathead sole 320-74
#> 87 10 Anarrhichthys ocellatus wolf-eel 320-73
#> 88 9 Pleurogrammus monopterygius Atka mackerel 320-74
#> 89 10 Lepidopsetta bilineata southern rock sole 320-73
#> 90 1 Arctoraja parmifera Alaska skate 324-73
#> 91 9 Atheresthes evermanni Kamchatka flounder 320-74
#> 92 2 Hippoglossoides elassodon flathead sole 325-76
#> 93 2 Myoxocephalus polyacanthocephalus great sculpin 325-76
#> 94 1 Pycnopodia helianthoides sunflower sea star 324-73
#> 95 2 Lepidopsetta bilineata southern rock sole 325-76
#> 96 2 Sebastes alutus Pacific ocean perch 325-76
#> 97 1 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 98 1 Bathyraja interrupta Bering skate 324-73
#> 99 2 Sebastes variabilis dusky rockfish 325-76
#> 100 2 Zaprora silenus prowfish 325-76
#> 101 10 Pleurogrammus monopterygius Atka mackerel 320-73
#> 102 2 Holothuroidea sea cucumber unid. 325-76
#> 103 1 Sebastes polyspinis northern rockfish 324-73
#> 104 1 Anoplopoma fimbria sablefish 324-73
#> 105 1 Hemilepidotus jordani yellow Irish lord 324-73
#> 106 2 Bathymaster signatus searcher 325-76
#> 107 10 Atheresthes stomias arrowtooth flounder 320-73
#> 108 1 Scyphozoa jellyfish unid. 324-73
#> 109 1 Thaliacea salp unid. 324-73
#> 110 1 Pagurus ochotensis Alaskan hermit 324-73
#> 111 1 Gorgonocephalus eucnemis basketstar 324-73
#> 112 1 Actiniaria sea anemone unid. 324-73
#> 113 2 Modiolus modiolus northern horsemussel 325-76
#> 114 9 Scyphozoa jellyfish unid. 320-74
#> 115 1 Triglops macellus roughspine sculpin 324-73
#> 116 2 Crystallichthys cyclospilus blotched snailfish 325-76
#> 117 2 Ceramaster patagonicus orange bat sea star 325-76
#> 118 1 Lepidopsetta polyxystra northern rock sole 324-73
#> 119 2 Gorgonocephalus eucnemis basketstar 325-76
#> 120 1 Sarritor frenatus sawback poacher 324-73
#> 121 2 Scyphozoa jellyfish unid. 325-76
#> 122 2 Elassochirus gilli Pacific red hermit 325-76
#> 123 2 Podothecus accipenserinus sturgeon poacher 325-76
#> 124 2 Nudibranchia nudibranch unid. 325-76
#> 125 1 Atheresthes evermanni Kamchatka flounder 324-73
#> 126 1 Oregonia gracilis graceful decorator crab 324-73
#> 127 1 Porifera sponge unid. 324-73
#> 128 2 Pagurus brandti sponge hermit 325-76
#> 129 2 Sarritor frenatus sawback poacher 325-76
#> 130 1 Crossaster papposus rose sea star 324-73
#> 131 1 Pagurus capillatus hairy hermit crab 324-73
#> 132 2 Ophiuroidea brittlestar unid. 325-76
#> 133 2 Elassochirus tenuimanus widehand hermit crab 325-76
#> 134 1 Ciliatocardium ciliatum hairy cockle 324-73
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 54 1616 1211.290 56195.947836 74971.84960
#> 55 1041 643.495 25096.701051 40599.64070
#> 56 489 399.642 15729.789050 19246.89308
#> 57 NA 322.619 14967.415313 0.00000
#> 58 357 98.418 3873.702911 14051.41274
#> 59 99 50.810 1981.621272 3861.06093
#> 60 88 45.950 2131.780006 4082.62547
#> 61 12 42.680 1980.073355 556.72166
#> 62 35 28.595 1115.222599 1365.02154
#> 63 9 26.500 1033.516310 351.00554
#> 64 33 23.050 1069.369513 1530.98455
#> 65 29 20.740 808.872765 1131.01785
#> 66 9 18.849 735.122601 351.00554
#> 67 1 18.780 732.431559 39.00062
#> 68 11 18.470 726.973651 432.95670
#> 69 40 17.560 684.850808 1560.02462
#> 70 6 15.540 611.649731 236.15820
#> 71 54 13.025 604.274964 2505.24745
#> 72 4 11.950 534.815361 179.01769
#> 73 1 11.130 516.359335 46.39347
#> 74 6 9.534 426.688674 268.52654
#> 75 17 9.350 433.778957 788.68901
#> 76 30 9.346 433.593383 1391.80414
#> 77 37 9.250 364.077221 1456.30888
#> 78 5 9.080 421.252719 231.96736
#> 79 14 8.980 350.225527 546.00862
#> 80 13 7.520 293.284628 507.00800
#> 81 9 7.370 287.434536 351.00554
#> 82 25 6.900 271.581927 983.99249
#> 83 24 6.170 242.849346 944.63279
#> 84 10 5.740 266.298525 463.93471
#> 85 1 5.380 209.823311 39.00062
#> 86 8 4.875 190.128000 312.00492
#> 87 1 4.670 209.003158 44.75442
#> 88 3 4.300 167.702647 117.00185
#> 89 7 4.100 183.493136 313.28096
#> 90 1 3.580 140.907724 39.35970
#> 91 3 2.940 114.661809 117.00185
#> 92 4 2.914 135.190575 185.57388
#> 93 1 2.720 126.190242 46.39347
#> 94 1 2.504 98.556688 39.35970
#> 95 8 2.259 104.802852 371.14777
#> 96 30 2.159 100.163505 1391.80414
#> 97 1 1.890 74.389832 39.35970
#> 98 1 1.850 72.815444 39.35970
#> 99 4 1.632 75.714145 185.57388
#> 100 1 1.592 73.858406 46.39347
#> 101 1 1.140 51.020043 44.75442
#> 102 3 0.994 46.115110 139.18041
#> 103 2 0.990 38.966103 78.71940
#> 104 2 0.974 38.336347 78.71940
#> 105 1 0.880 34.636536 39.35970
#> 106 2 0.756 35.073464 92.78694
#> 107 2 0.730 32.670729 89.50885
#> 108 4 0.576 22.671187 157.43880
#> 109 5 0.452 17.790584 196.79850
#> 110 15 0.442 17.396987 590.39549
#> 111 1 0.430 16.924671 39.35970
#> 112 4 0.394 15.507722 157.43880
#> 113 12 0.375 17.397552 556.72166
#> 114 1 0.295 11.505182 39.00062
#> 115 5 0.292 11.493032 196.79850
#> 116 1 0.222 10.299351 46.39347
#> 117 4 0.200 9.278694 185.57388
#> 118 1 0.174 6.848588 39.35970
#> 119 2 0.145 6.727053 92.78694
#> 120 3 0.140 5.510358 118.07910
#> 121 2 0.095 4.407380 92.78694
#> 122 2 0.085 3.943445 92.78694
#> 123 1 0.072 3.340330 46.39347
#> 124 2 0.055 2.551641 92.78694
#> 125 1 0.042 1.653107 39.35970
#> 126 1 0.042 1.653107 39.35970
#> 127 NA 0.033 1.298870 0.00000
#> 128 2 0.030 1.391804 92.78694
#> 129 1 0.024 1.113443 46.39347
#> 130 1 0.014 0.551036 39.35970
#> 131 1 0.012 0.472316 39.35970
#> 132 2 0.005 0.231967 92.78694
#> 133 1 0.005 0.231967 46.39347
#> 134 1 0.002 0.078719 39.35970
#>
#> $catch$`2022`
#> haul scientific_name common_name station
#> 13 6 Gadus chalcogrammus walleye pollock 324-73
#> 14 5 Gadus chalcogrammus walleye pollock 326-76
#> 15 5 Atheresthes stomias arrowtooth flounder 326-76
#> 16 6 Atheresthes stomias arrowtooth flounder 324-73
#> 17 5 Gadus macrocephalus Pacific cod 326-76
#> 18 5 Hippoglossoides elassodon flathead sole 326-76
#> 19 6 Hippoglossus stenolepis Pacific halibut 324-73
#> 20 6 Enteroctopus dofleini giant octopus 324-73
#> 21 6 Hippoglossoides elassodon flathead sole 324-73
#> 22 5 Hemilepidotus jordani yellow Irish lord 326-76
#> 23 5 Lepidopsetta polyxystra northern rock sole 326-76
#> 24 6 Beringraja binoculata big skate 324-73
#> 25 6 Parophrys vetulus English sole 324-73
#> 26 5 Hippoglossus stenolepis Pacific halibut 326-76
#> 27 6 Glyptocephalus zachirus rex sole 324-73
#> 28 6 Chionoecetes bairdi Tanner crab 324-73
#> 29 6 Pycnopodia helianthoides sunflower sea star 324-73
#> 30 6 Myoxocephalus polyacanthocephalus great sculpin 324-73
#> 31 6 Gadus macrocephalus Pacific cod 324-73
#> 32 5 Myoxocephalus polyacanthocephalus great sculpin 326-76
#> 33 6 Lepidopsetta bilineata southern rock sole 324-73
#> 34 6 Hemilepidotus jordani yellow Irish lord 324-73
#> 35 5 Pleurogrammus monopterygius Atka mackerel 326-76
#> 36 6 Lepidopsetta polyxystra northern rock sole 324-73
#> 37 5 Atheresthes evermanni Kamchatka flounder 326-76
#> 38 6 Microstomus pacificus Dover sole 324-73
#> 39 5 Sebastes alutus Pacific ocean perch 326-76
#> 40 5 Malacocottus zonurus darkfin sculpin 326-76
#> 41 5 Microstomus pacificus Dover sole 326-76
#> 42 5 Sebastes polyspinis northern rockfish 326-76
#> 43 6 Gorgonocephalus eucnemis basketstar 324-73
#> 44 6 Pennatuloidea sea whip or sea pen unid. 324-73
#> 45 6 Porifera sponge unid. 324-73
#> 46 5 Actiniaria sea anemone unid. 326-76
#> 47 5 Triglops forficatus scissortail sculpin 326-76
#> 48 5 Triglops scepticus spectacled sculpin 326-76
#> 49 6 Pagurus trigonocheirus fuzzy hermit crab 324-73
#> 50 5 Modiolus modiolus northern horsemussel 326-76
#> 51 6 Pagurus confragosus knobbyhand hermit 324-73
#> 52 6 Ascidiacea tunicate unid. 324-73
#> 53 6 Triglops macellus roughspine sculpin 324-73
#> count weight_kg cpue_kgkm2 cpue_nokm2
#> 13 1066 715.400 25276.726092 37664.22982
#> 14 620 569.090 22754.334435 24789.90555
#> 15 295 157.540 6299.035033 11795.19700
#> 16 201 134.890 4765.973697 7101.79193
#> 17 47 103.320 4131.117809 1879.23478
#> 18 104 55.660 2225.493779 4158.30674
#> 19 33 50.280 1776.507951 1165.96584
#> 20 2 24.500 865.641305 70.66460
#> 21 96 21.030 743.038230 3391.90062
#> 22 24 18.960 758.091305 959.60925
#> 23 37 16.840 673.325822 1479.39759
#> 24 1 15.560 549.770559 35.33230
#> 25 18 13.171 465.361699 635.98137
#> 26 6 8.480 339.061934 239.90231
#> 27 26 7.860 277.711863 918.63975
#> 28 25 6.400 226.126708 883.30745
#> 29 2 6.300 222.593478 70.66460
#> 30 2 4.865 171.891630 70.66460
#> 31 4 3.870 136.735994 141.32919
#> 32 1 3.085 123.349772 39.98372
#> 33 4 2.975 105.113587 141.32919
#> 34 5 2.940 103.876957 176.66149
#> 35 2 1.980 79.167763 79.96744
#> 36 2 1.455 51.408494 70.66460
#> 37 4 1.380 55.177532 159.93488
#> 38 5 1.280 45.225342 176.66149
#> 39 4 1.105 44.182009 159.93488
#> 40 2 0.975 38.984126 79.96744
#> 41 3 0.915 36.585103 119.95116
#> 42 1 0.860 34.385998 39.98372
#> 43 2 0.835 29.502469 70.66460
#> 44 2 0.480 16.959503 70.66460
#> 45 NA 0.170 6.006491 0.00000
#> 46 1 0.165 6.597314 39.98372
#> 47 1 0.100 3.998372 39.98372
#> 48 2 0.085 3.398616 79.96744
#> 49 1 0.065 2.296599 35.33230
#> 50 1 0.060 2.399023 39.98372
#> 51 1 0.055 1.943276 35.33230
#> 52 NA 0.040 1.413292 0.00000
#> 53 1 0.020 0.706646 35.33230
#>
#> $catch$`2024`
#> haul scientific_name common_name station count
#> 1 6 Atheresthes stomias arrowtooth flounder 321-74 295
#> 2 6 Lepidopsetta bilineata southern rock sole 321-74 156
#> 3 6 Gadus macrocephalus Pacific cod 321-74 11
#> 4 6 Hippoglossus stenolepis Pacific halibut 321-74 18
#> 5 6 Beringraja rhina longnose skate 321-74 1
#> 6 6 Hippoglossoides elassodon flathead sole 321-74 27
#> 7 6 Gadus chalcogrammus walleye pollock 321-74 6
#> 8 6 Scyphozoa jellyfish unid. 321-74 10
#> 9 6 Lepidopsetta polyxystra northern rock sole 321-74 5
#> 10 6 Atheresthes evermanni Kamchatka flounder 321-74 1
#> 11 6 Hydroidolina hydroid unid. 321-74 NA
#> 12 6 Leptocottus armatus Pacific staghorn sculpin 321-74 1
#> weight_kg cpue_kgkm2 cpue_nokm2
#> 1 123.970 3849.731834 9160.85255
#> 2 95.986 2980.724045 4844.38304
#> 3 20.960 650.886337 341.59111
#> 4 19.194 596.045437 558.96727
#> 5 18.650 579.152204 31.05374
#> 6 15.764 489.531117 838.45091
#> 7 7.780 241.598078 186.32243
#> 8 3.396 105.458492 310.53737
#> 9 2.170 67.386610 155.26869
#> 10 0.410 12.732032 31.05374
#> 11 0.360 11.179345 0.00000
#> 12 0.152 4.720168 31.05374
#>
#>
#> $catch_means
#> scientific_name common_name station count
#> 1 Pleurogrammus monopterygius Atka mackerel 326-76 1711.0
#> 2 Gadus chalcogrammus walleye pollock 325-76 1234.3
#> 3 Gadus chalcogrammus walleye pollock 321-74 853.8
#> 4 Gadus chalcogrammus walleye pollock 324-73 404.9
#> 5 Porifera sponge unid. 325-76 NaN
#> 6 Gadus chalcogrammus walleye pollock 326-76 178.5
#> 7 Atheresthes stomias arrowtooth flounder 320-74 221.2
#> 8 Lepidopsetta bilineata southern rock sole 321-74 141.8
#> 9 Lepidopsetta polyxystra northern rock sole 320-73 150.2
#> 10 Atheresthes stomias arrowtooth flounder 321-74 176.7
#> 11 Gadus macrocephalus Pacific cod 325-76 23.0
#> 12 Gadus macrocephalus Pacific cod 320-73 47.3
#> 13 Lepidopsetta bilineata southern rock sole 320-73 78.8
#> 14 Gadus macrocephalus Pacific cod 326-76 30.3
#> 15 Atheresthes stomias arrowtooth flounder 324-73 206.1
#> 16 Pleurogrammus monopterygius Atka mackerel 320-74 40.8
#> 17 Sebastes polyspinis northern rockfish 325-76 88.0
#> 18 Gadus macrocephalus Pacific cod 320-74 22.0
#> 19 Lepidopsetta polyxystra northern rock sole 325-76 108.7
#> 20 Lepidopsetta polyxystra northern rock sole 320-74 84.3
#> 21 Hippoglossus stenolepis Pacific halibut 320-74 28.9
#> 22 Atheresthes stomias arrowtooth flounder 326-76 79.3
#> 23 Hippoglossus stenolepis Pacific halibut 325-76 28.7
#> 24 Hippoglossus stenolepis Pacific halibut 320-73 41.8
#> 25 Hemilepidotus jordani yellow Irish lord 325-76 33.0
#> 26 Gadus macrocephalus Pacific cod 321-74 15.3
#> 27 Somniosus pacificus Pacific sleeper shark 324-73 1.0
#> 28 Hippoglossus stenolepis Pacific halibut 321-74 22.0
#> 29 Lepidopsetta bilineata southern rock sole 320-74 31.0
#> 30 Hippoglossus stenolepis Pacific halibut 324-73 14.7
#> 31 Hippoglossoides elassodon flathead sole 324-73 122.6
#> 32 Pleurogrammus monopterygius Atka mackerel 325-76 18.0
#> 33 Lepidopsetta polyxystra northern rock sole 326-76 46.0
#> 34 Hippoglossoides elassodon flathead sole 321-74 32.5
#> 35 Hippoglossus stenolepis Pacific halibut 326-76 12.3
#> 36 Hippoglossoides elassodon flathead sole 325-76 27.0
#> 37 Bathyraja aleutica Aleutian skate 320-74 1.0
#> 38 Atheresthes stomias arrowtooth flounder 325-76 29.7
#> 39 Hippoglossoides elassodon flathead sole 326-76 28.3
#> 40 Porifera sponge unid. 326-76 1.0
#> 41 Enteroctopus dofleini giant octopus 320-74 2.0
#> 42 Beringraja rhina longnose skate 321-74 1.0
#> 43 Beringraja binoculata big skate 324-73 1.0
#> 44 Hemilepidotus jordani yellow Irish lord 326-76 19.0
#> 45 Enteroctopus dofleini giant octopus 324-73 1.2
#> 46 Lepidopsetta bilineata southern rock sole 325-76 18.0
#> 47 Lepidopsetta polyxystra northern rock sole 321-74 24.2
#> 48 Enteroctopus dofleini giant octopus 325-76 1.0
#> 49 Hippoglossoides elassodon flathead sole 320-74 14.4
#> 50 Gadus macrocephalus Pacific cod 324-73 7.6
#> 51 Atheresthes stomias arrowtooth flounder 320-73 17.5
#> 52 Enteroctopus dofleini giant octopus 321-74 1.0
#> 53 Glyptocephalus zachirus rex sole 324-73 33.7
#> 54 Sebastes alutus Pacific ocean perch 320-74 24.0
#> 55 Sebastes variabilis dusky rockfish 320-74 12.0
#> 56 Parophrys vetulus English sole 324-73 12.0
#> 57 Chionoecetes bairdi Tanner crab 324-73 21.5
#> 58 Gadus chalcogrammus walleye pollock 320-74 8.7
#> 59 Hemilepidotus jordani yellow Irish lord 320-74 9.8
#> 60 Glyptocephalus zachirus rex sole 320-74 10.3
#> 61 Sebastes polyspinis northern rockfish 320-74 11.0
#> 62 Arctoraja parmifera Alaska skate 325-76 1.0
#> 63 Hexagrammos decagrammus kelp greenling 325-76 14.7
#> 64 Myoxocephalus polyacanthocephalus great sculpin 320-73 1.0
#> 65 Anarrhichthys ocellatus wolf-eel 320-73 1.0
#> 66 Anarrhichthys ocellatus wolf-eel 320-74 1.0
#> 67 Actiniaria sea anemone unid. 321-74 21.3
#> 68 Myoxocephalus polyacanthocephalus great sculpin 320-74 1.5
#> 69 Clupea pallasii Pacific herring 320-73 10.0
#> 70 Arctoraja parmifera Alaska skate 324-73 1.0
#> 71 Pycnopodia helianthoides sunflower sea star 324-73 1.5
#> 72 Myoxocephalus polyacanthocephalus great sculpin 324-73 1.6
#> 73 Oncorhynchus keta chum salmon 325-76 1.0
#> 74 Anoplopoma fimbria sablefish 324-73 4.7
#> 75 Lepidopsetta bilineata southern rock sole 324-73 3.0
#> 76 Bathymaster signatus searcher 325-76 10.0
#> 77 Myoxocephalus polyacanthocephalus great sculpin 325-76 1.0
#> 78 Pleurogrammus monopterygius Atka mackerel 320-73 4.0
#> 79 Myoxocephalus polyacanthocephalus great sculpin 326-76 1.0
#> 80 Atheresthes evermanni Kamchatka flounder 320-74 5.5
#> 81 Lepidopsetta bilineata southern rock sole 326-76 3.2
#> 82 Pleurogrammus monopterygius Atka mackerel 324-73 3.0
#> 83 Hemilepidotus jordani yellow Irish lord 321-74 2.0
#> 84 Hemilepidotus jordani yellow Irish lord 324-73 3.3
#> 85 Pleuronectes quadrituberculatus Alaska plaice 324-73 1.0
#> 86 Sebastes variabilis dusky rockfish 325-76 4.0
#> 87 Zaprora silenus prowfish 325-76 1.0
#> 88 Pleurogrammus monopterygius Atka mackerel 321-74 2.0
#> 89 Bathyraja interrupta Bering skate 324-73 1.0
#> 90 Bathyraja taranetzi mud skate 320-74 1.0
#> 91 Porifera sponge unid. 321-74 NaN
#> 92 Hexagrammos decagrammus kelp greenling 320-74 3.0
#> 93 Hemitripterus bolini bigmouth sculpin 326-76 1.3
#> 94 Hemilepidotus jordani yellow Irish lord 320-73 1.7
#> 95 Aptocyclus ventricosus smooth lumpsucker 320-73 1.0
#> 96 Lethasterias nanimensis blackspined sea star 325-76 10.0
#> 97 Berryteuthis magister magistrate armhook squid 321-74 5.0
#> 98 Sebastes ciliatus dark rockfish 321-74 1.0
#> 99 Pycnopodia helianthoides sunflower sea star 320-74 1.0
#> 100 Sebastes alutus Pacific ocean perch 325-76 15.3
#> 101 Zoanthidae sp. A hot dog zoanthid 325-76 NaN
#> 102 Gymnocanthus galeatus armorhead sculpin 324-73 5.3
#> 103 Aptocyclus ventricosus smooth lumpsucker 320-74 1.0
#> 104 Holothuroidea sea cucumber unid. 325-76 2.5
#> 105 Sebastes variabilis dusky rockfish 321-74 1.0
#> 106 Limanda aspera yellowfin sole 321-74 1.0
#> 107 Glyptocephalus zachirus rex sole 321-74 1.0
#> 108 Gadus chalcogrammus walleye pollock 320-73 1.7
#> 109 Lethasterias nanimensis blackspined sea star 320-73 3.0
#> 110 Hippoglossoides elassodon flathead sole 320-73 1.0
#> 111 Malacocottus zonurus darkfin sculpin 326-76 2.0
#> 112 Scyphozoa jellyfish unid. 320-73 3.0
#> 113 Microstomus pacificus Dover sole 326-76 3.0
#> 114 Scyphozoa jellyfish unid. 321-74 4.0
#> 115 Lepidopsetta polyxystra northern rock sole 324-73 1.6
#> 116 Sebastes melanostictus blackspotted rockfish 320-74 1.0
#> 117 Malacocottus zonurus darkfin sculpin 320-74 1.0
#> 118 Sebastes polyspinis northern rockfish 326-76 1.0
#> 119 Atheresthes evermanni Kamchatka flounder 326-76 2.0
#> 120 Sebastes alutus Pacific ocean perch 324-73 8.0
#> 121 Scyphozoa jellyfish unid. 320-74 1.7
#> 122 Gorgonocephalus eucnemis basketstar 324-73 2.5
#> 123 Sebastes variabilis dusky rockfish 324-73 2.0
#> 124 Thaliacea salp unid. 321-74 NaN
#> 125 Pagurus brandti sponge hermit 325-76 15.5
#> 126 Sebastes alutus Pacific ocean perch 326-76 3.0
#> 127 Microstomus pacificus Dover sole 324-73 3.0
#> 128 Podothecus accipenserinus sturgeon poacher 324-73 8.4
#> 129 Thaliacea salp unid. 324-73 5.0
#> 130 Ascidiacea tunicate unid. 326-76 11.0
#> 131 Gymnocanthus galeatus armorhead sculpin 325-76 2.0
#> 132 Gymnocanthus galeatus armorhead sculpin 326-76 2.0
#> 133 Atheresthes evermanni Kamchatka flounder 320-73 2.0
#> 134 Actiniaria sea anemone unid. 325-76 19.0
#> 135 Berryteuthis magister magistrate armhook squid 320-74 2.0
#> 136 Actiniaria sea anemone unid. 320-74 3.8
#> 137 Sebastes variegatus harlequin rockfish 326-76 1.0
#> 138 Triglops forficatus scissortail sculpin 326-76 4.0
#> 139 Pagurus ochotensis Alaskan hermit 324-73 15.0
#> 140 Berryteuthis magister magistrate armhook squid 326-76 1.5
#> 141 Hemilepidotus hemilepidotus red Irish lord 320-73 1.0
#> 142 Pennatuloidea sea whip or sea pen unid. 324-73 2.0
#> 143 Scyphozoa jellyfish unid. 324-73 2.0
#> 144 Gorgonocephalus eucnemis basketstar 321-74 3.5
#> 145 Atheresthes evermanni Kamchatka flounder 324-73 4.0
#> 146 Modiolus modiolus northern horsemussel 325-76 10.5
#> 147 Porifera sponge unid. 320-74 1.0
#> 148 Crossaster papposus rose sea star 325-76 8.0
#> 149 Sebastes polyspinis northern rockfish 324-73 1.2
#> 150 Clupea pallasii Pacific herring 324-73 1.0
#> 151 Lethasterias nanimensis blackspined sea star 324-73 3.0
#> 152 Chionoecetes bairdi Tanner crab 321-74 2.0
#> 153 Porifera sponge unid. 324-73 1.0
#> 154 Thaliacea salp unid. 320-73 NaN
#> 155 Polychaeta polychaete worm unid. 321-74 NaN
#> 156 Hydroidolina hydroid unid. 321-74 NaN
#> 157 Lethasterias nanimensis blackspined sea star 320-74 1.0
#> 158 Mediaster aequalis vermilion sea star 325-76 4.0
#> 159 Sebastes ciliatus dark rockfish 325-76 1.0
#> 160 Crystallichthys cyclospilus blotched snailfish 325-76 1.0
#> 161 Gorgonocephalus eucnemis basketstar 326-76 1.0
#> 162 Ascidiacea tunicate unid. 320-73 1.0
#> 163 Gymnocanthus galeatus armorhead sculpin 321-74 1.5
#> 164 Ceramaster patagonicus orange bat sea star 325-76 4.0
#> 165 Atheresthes evermanni Kamchatka flounder 321-74 2.5
#> 166 Pagurus trigonocheirus fuzzy hermit crab 324-73 1.0
#> 167 Pagurus trigonocheirus fuzzy hermit crab 320-74 5.0
#> 168 Elassochirus cavimanus purple hermit 325-76 5.0
#> 169 Scyphozoa jellyfish unid. 326-76 1.0
#> 170 Actiniaria sea anemone unid. 324-73 2.5
#> 171 Crystallichthys cyclospilus blotched snailfish 320-74 1.0
#> 172 Modiolus modiolus northern horsemussel 326-76 2.0
#> 173 Sebastes ciliatus dark rockfish 320-74 1.0
#> 174 Ceramaster japonicus red bat star 325-76 3.0
#> 175 Cheiraster dawsoni fragile sea star 325-76 11.0
#> 176 Pennatuloidea sea whip or sea pen unid. 320-73 NaN
#> 177 Pagurus brandti sponge hermit 320-74 9.0
#> 178 Triglops forficatus scissortail sculpin 325-76 5.0
#> 179 Pagurus brandti sponge hermit 324-73 8.0
#> 180 Gorgonocephalus eucnemis basketstar 320-74 1.0
#> 181 Gorgonocephalus eucnemis basketstar 325-76 2.0
#> 182 Holothuroidea sea cucumber unid. 320-74 4.2
#> 183 Pagurus cornutus hornyhand hermit 320-74 5.0
#> 184 Acantholithodes hispidus fuzzy crab 325-76 4.0
#> 185 Bathymaster signatus searcher 324-73 1.0
#> 186 Pagurus ochotensis Alaskan hermit 321-74 2.5
#> 187 Erimacrus isenbeckii horsehair crab 324-73 1.0
#> 188 Triglops macellus roughspine sculpin 324-73 3.0
#> 189 Elassochirus cavimanus purple hermit 320-74 5.3
#> 190 Bathymaster signatus searcher 326-76 1.0
#> 191 Thaliacea salp unid. 320-74 NaN
#> 192 Echinarachnius parma parma sand dollar 321-74 4.0
#> 193 Sarritor frenatus sawback poacher 324-73 3.0
#> 194 Solaster dawsoni morning sun sea star 320-74 1.0
#> 195 Elassochirus cavimanus purple hermit 321-74 2.5
#> 196 Leptocottus armatus Pacific staghorn sculpin 321-74 1.0
#> 197 Gymnocanthus galeatus armorhead sculpin 320-74 2.0
#> 198 Polychaeta polychaete worm unid. 324-73 1.0
#> 199 Actiniaria sea anemone unid. 326-76 1.5
#> 200 Scyphozoa jellyfish unid. 325-76 2.0
#> 201 Crystallichthys cyclospilus blotched snailfish 326-76 1.0
#> 202 Elassochirus gilli Pacific red hermit 325-76 2.0
#> 203 Polyplacophora chiton unid. 324-73 1.0
#> 204 Pagurus confragosus knobbyhand hermit 324-73 3.0
#> 205 Modiolus modiolus northern horsemussel 320-74 1.0
#> 206 Podothecus accipenserinus sturgeon poacher 321-74 1.2
#> 207 Pagurus confragosus knobbyhand hermit 320-74 4.5
#> 208 Pagurus aleuticus Aleutian hermit 324-73 2.8
#> 209 Ammodytes sp. sand lance unid. 320-73 2.0
#> 210 Podothecus accipenserinus sturgeon poacher 325-76 1.0
#> 211 Thaliacea salp unid. 326-76 1.0
#> 212 Elassochirus cavimanus purple hermit 326-76 2.0
#> 213 Triglops scepticus spectacled sculpin 320-74 3.0
#> 214 Bryozoa bryozoan unid. 326-76 NaN
#> 215 Triglops macellus roughspine sculpin 321-74 1.0
#> 216 Podothecus accipenserinus sturgeon poacher 320-73 1.0
#> 217 Ceramaster japonicus red bat star 326-76 1.0
#> 218 Elassochirus tenuimanus widehand hermit crab 326-76 2.0
#> 219 Lethasterias nanimensis blackspined sea star 321-74 1.0
#> 220 Diplopteraster multipes pincushion sea star 326-76 1.0
#> 221 Ascidiacea tunicate unid. 325-76 1.0
#> 222 Pseudarchaster parelii scarlet sea star 325-76 2.0
#> 223 Ceramaster patagonicus orange bat sea star 320-74 1.0
#> 224 Ascidiacea tunicate unid. 321-74 1.0
#> 225 Ophiuroidea brittlestar unid. 320-74 8.2
#> 226 Pagurus confragosus knobbyhand hermit 326-76 1.0
#> 227 Ascidiacea tunicate unid. 324-73 NaN
#> 228 Elassochirus tenuimanus widehand hermit crab 321-74 1.5
#> 229 Gorgonocephalus eucnemis basketstar 320-73 1.0
#> 230 Triglops scepticus spectacled sculpin 326-76 1.5
#> 231 Holothuroidea sea cucumber unid. 326-76 1.0
#> 232 Pennatuloidea sea whip or sea pen unid. 326-76 1.0
#> 233 Diplopteraster multipes pincushion sea star 320-74 1.0
#> 234 Sarritor frenatus sawback poacher 326-76 1.0
#> 235 Hyas lyratus Pacific lyre crab 326-76 2.0
#> 236 Polychaeta polychaete worm unid. 325-76 NaN
#> 237 Pagurus aleuticus Aleutian hermit 325-76 2.0
#> 238 Elassochirus tenuimanus widehand hermit crab 320-74 2.5
#> 239 Crossaster papposus rose sea star 324-73 1.0
#> 240 Crossaster papposus rose sea star 321-74 1.0
#> 241 Hyas lyratus Pacific lyre crab 320-74 2.8
#> 242 Oregonia gracilis graceful decorator crab 324-73 1.0
#> 243 Pseudarchaster parelii scarlet sea star 326-76 1.0
#> 244 Pagurus ochotensis Alaskan hermit 326-76 2.0
#> 245 Ophiuroidea brittlestar unid. 321-74 9.7
#> 246 Rhinolithodes wosnessenskii rhinoceros crab 325-76 1.0
#> 247 Pseudarchaster parelii scarlet sea star 324-73 1.0
#> 248 Nudibranchia nudibranch unid. 324-73 1.0
#> 249 Ascidiacea tunicate unid. 320-74 2.0
#> 250 Hyas lyratus Pacific lyre crab 321-74 1.0
#> 251 Lethasterias nanimensis blackspined sea star 326-76 1.0
#> 252 Nudibranchia nudibranch unid. 325-76 1.5
#> 253 Pagurus aleuticus Aleutian hermit 320-74 1.0
#> 254 Cheiraster dawsoni fragile sea star 321-74 1.0
#> 255 Nudibranchia nudibranch unid. 326-76 1.0
#> 256 Rajiformes egg case skate egg case unid. 320-74 4.0
#> 257 Ammodytes sp. sand lance unid. 320-74 1.0
#> 258 Ceramaster patagonicus orange bat sea star 326-76 1.0
#> 259 Sarritor frenatus sawback poacher 325-76 1.0
#> 260 Bryozoa bryozoan unid. 320-74 NaN
#> 261 Pseudarchaster parelii scarlet sea star 321-74 1.0
#> 262 Cheiraster dawsoni fragile sea star 320-74 2.0
#> 263 Aptocyclus ventricosus smooth lumpsucker 326-76 3.0
#> 264 Modiolus modiolus northern horsemussel 321-74 1.0
#> 265 Rajiformes egg case skate egg case unid. 324-73 NaN
#> 266 Leptychaster arcticus North Pacific sea star 325-76 1.0
#> 267 Castaneobuccinum castaneum chestnut whelk 320-74 1.0
#> 268 Ophiuroidea brittlestar unid. 320-73 3.0
#> 269 Pagurus aleuticus Aleutian hermit 326-76 1.0
#> 270 Elassochirus tenuimanus widehand hermit crab 325-76 1.0
#> 271 Pagurus brandti sponge hermit 321-74 1.5
#> 272 Bryozoa bryozoan unid. 325-76 NaN
#> 273 Ceramaster arcticus Arctic bat sea star 320-74 1.0
#> 274 Polychaeta polychaete worm unid. 320-74 1.5
#> 275 Porifera sponge unid. 320-73 NaN
#> 276 Pagurus aleuticus Aleutian hermit 321-74 1.0
#> 277 Pedicellaster magister majestic sea star 326-76 1.0
#> 278 Pagurus capillatus hairy hermit crab 324-73 1.0
#> 279 Ctenodiscus crispatus common mud star 321-74 1.0
#> 280 Pedicellaster magister majestic sea star 325-76 2.0
#> 281 Brachiopoda lampshell unid. 326-76 1.0
#> 282 Cheiraster dawsoni fragile sea star 326-76 1.0
#> 283 Eumicrotremus sp. spiny lumpsuckers 326-76 2.0
#> 284 Ophiuroidea brittlestar unid. 326-76 3.0
#> 285 Hyas lyratus Pacific lyre crab 324-73 1.0
#> 286 Cheiraster dawsoni fragile sea star 324-73 1.0
#> 287 Ophiuroidea brittlestar unid. 325-76 3.0
#> 288 Pagurus brandti sponge hermit 320-73 1.0
#> 289 Pagurus rathbuni longfinger hermit 324-73 1.0
#> 290 Pagurus dalli whiteknee hermit 326-76 1.0
#> 291 Labidochirus splendescens splendid hermit 324-73 1.0
#> 292 Keenocardium blandum low-rib cockle 320-74 1.0
#> 293 Leptasterias hylodes Aleutian sea star 325-76 2.0
#> 294 Labidochirus splendescens splendid hermit 320-74 1.0
#> 295 Isopoda isopod unid. 326-76 1.0
#> 296 Bulbus fragilis fragile moonsnail 320-73 1.0
#> 297 Hirudinea leech unid. 320-73 1.0
#> 298 Rhamphocottus richardsonii grunt sculpin 321-74 1.0
#> 299 Hydroidolina hydroid unid. 326-76 NaN
#> 300 Oregonia gracilis graceful decorator crab 325-76 1.0
#> 301 Bryozoa bryozoan unid. 321-74 NaN
#> 302 Paguridae hermit crab unid. 320-74 3.0
#> 303 Ciliatocardium ciliatum hairy cockle 324-73 1.0
#> weight_kg cpue_kgkm2 cpue_nokm2 Freq
#> 1 2044.63 108443.71 91810.95 24
#> 2 1251.75 55114.50 54838.15 31
#> 3 784.48 35483.43 38640.10 31
#> 4 349.85 13497.17 15445.25 31
#> 5 236.41 10870.82 0.00 23
#> 6 175.00 7327.82 7455.60 31
#> 7 151.60 6297.82 9178.62 37
#> 8 113.58 4745.90 5826.77 33
#> 9 77.45 3416.72 6626.83 35
#> 10 83.37 3215.80 6766.89 37
#> 11 70.91 3113.01 1012.85 39
#> 12 69.07 2993.11 2049.81 39
#> 13 57.65 2582.22 3502.65 33
#> 14 55.44 2532.10 1371.18 39
#> 15 63.17 2461.77 8242.56 37
#> 16 60.12 2446.28 1661.49 24
#> 17 45.95 2131.78 4082.63 10
#> 18 43.21 1917.41 970.52 39
#> 19 42.69 1885.36 4838.62 35
#> 20 42.58 1838.95 3664.70 35
#> 21 40.92 1820.15 1295.25 39
#> 22 42.31 1765.77 3320.51 37
#> 23 38.12 1681.20 1245.72 39
#> 24 37.27 1648.29 1844.99 39
#> 25 26.45 1188.56 1487.43 31
#> 26 28.52 1168.98 619.84 39
#> 27 25.80 1108.64 42.97 1
#> 28 25.83 1086.57 927.17 39
#> 29 23.82 1070.78 1396.34 33
#> 30 25.15 999.26 579.23 39
#> 31 24.10 967.45 4931.24 30
#> 32 21.93 962.55 799.10 24
#> 33 21.20 933.23 2034.59 35
#> 34 22.35 905.39 1315.20 30
#> 35 17.20 844.16 558.42 39
#> 36 18.41 797.25 1170.00 30
#> 37 18.78 732.43 39.00 1
#> 38 15.60 706.91 1346.77 37
#> 39 15.69 654.63 1180.18 30
#> 40 11.08 607.04 11.04 23
#> 41 14.03 580.53 83.59 9
#> 42 18.65 579.15 31.05 1
#> 43 15.41 569.75 36.99 2
#> 44 12.67 552.17 834.00 31
#> 45 13.81 537.63 48.98 9
#> 46 12.20 534.53 794.72 33
#> 47 12.42 518.97 999.75 35
#> 48 11.13 516.36 46.39 9
#> 49 11.54 511.25 635.90 30
#> 50 11.72 483.14 312.10 39
#> 51 10.06 460.45 821.13 37
#> 52 10.25 428.98 41.85 9
#> 53 10.42 422.03 1363.23 14
#> 54 10.62 416.61 941.29 10
#> 55 9.85 402.84 490.12 6
#> 56 10.09 375.29 440.23 2
#> 57 8.46 348.97 868.96 7
#> 58 8.53 346.21 351.71 31
#> 59 7.06 298.55 414.56 31
#> 60 6.59 282.28 443.79 14
#> 61 7.00 279.66 440.75 10
#> 62 5.98 274.83 45.96 4
#> 63 5.45 248.30 673.30 5
#> 64 4.46 233.08 52.31 12
#> 65 5.28 231.61 43.92 3
#> 66 4.48 202.69 45.24 3
#> 67 4.50 194.03 931.06 16
#> 68 4.20 189.18 67.49 12
#> 69 4.32 186.14 430.87 2
#> 70 4.57 182.07 40.21 4
#> 71 4.40 160.58 55.01 3
#> 72 3.94 157.43 62.41 12
#> 73 3.12 143.39 45.96 1
#> 74 3.40 137.83 188.52 3
#> 75 3.17 126.83 118.19 33
#> 76 2.75 126.46 460.01 4
#> 77 2.72 126.19 46.39 12
#> 78 2.81 125.57 181.32 24
#> 79 3.08 123.35 39.98 12
#> 80 2.81 115.01 231.92 15
#> 81 2.43 107.66 143.95 33
#> 82 2.53 97.77 115.94 24
#> 83 2.25 91.84 82.00 31
#> 84 2.08 81.73 131.43 31
#> 85 2.03 78.61 38.65 1
#> 86 1.63 75.71 185.57 6
#> 87 1.59 73.86 46.39 1
#> 88 1.67 72.86 86.28 24
#> 89 1.85 72.82 39.36 1
#> 90 1.72 69.43 40.32 1
#> 91 1.64 68.73 0.00 23
#> 92 1.48 67.66 135.87 5
#> 93 1.30 67.22 65.20 3
#> 94 1.38 65.32 81.23 31
#> 95 1.41 63.18 44.68 3
#> 96 1.35 61.95 459.58 6
#> 97 1.37 60.49 220.43 5
#> 98 1.33 60.04 45.28 3
#> 99 1.32 59.41 45.01 3
#> 100 1.30 59.24 699.86 10
#> 101 1.23 56.53 0.00 1
#> 102 1.33 56.12 224.49 10
#> 103 1.11 50.22 45.24 3
#> 104 1.08 49.94 115.55 7
#> 105 1.28 46.23 36.06 6
#> 106 1.00 45.10 45.28 1
#> 107 0.93 43.59 46.87 14
#> 108 0.86 42.73 81.76 31
#> 109 0.79 41.11 156.92 6
#> 110 0.95 40.76 43.09 30
#> 111 0.98 38.98 79.97 2
#> 112 0.72 37.16 78.46 16
#> 113 0.92 36.59 119.95 3
#> 114 1.17 36.53 129.48 16
#> 115 0.84 33.36 64.41 35
#> 116 0.77 31.05 40.32 1
#> 117 0.73 29.51 40.32 2
#> 118 0.65 29.40 47.60 10
#> 119 0.67 29.24 87.78 15
#> 120 0.68 28.91 341.10 10
#> 121 0.61 27.61 56.28 16
#> 122 0.70 27.46 99.67 11
#> 123 0.63 27.08 85.44 6
#> 124 0.70 27.05 0.00 10
#> 125 0.59 27.03 712.78 8
#> 126 0.58 26.95 141.65 10
#> 127 0.74 26.36 107.65 3
#> 128 0.63 25.69 345.95 11
#> 129 0.62 25.21 65.60 10
#> 130 0.57 25.10 489.19 11
#> 131 0.52 23.07 88.85 10
#> 132 0.51 22.62 89.03 10
#> 133 0.52 22.50 87.36 15
#> 134 0.45 20.86 873.19 16
#> 135 0.44 19.72 89.61 5
#> 136 0.42 19.44 174.13 16
#> 137 0.32 17.89 55.22 1
#> 138 0.38 17.84 187.43 6
#> 139 0.44 17.40 590.40 5
#> 140 0.38 17.18 68.03 5
#> 141 0.39 17.04 43.68 1
#> 142 0.48 16.96 70.66 4
#> 143 0.40 15.98 79.90 16
#> 144 0.34 15.69 162.66 11
#> 145 0.37 15.33 166.30 15
#> 146 0.32 14.90 485.17 9
#> 147 0.32 14.50 16.90 23
#> 148 0.31 14.43 367.66 5
#> 149 0.36 14.39 51.27 10
#> 150 0.37 14.14 38.65 2
#> 151 0.34 14.08 125.67 6
#> 152 0.30 13.87 93.74 7
#> 153 0.33 13.69 7.16 23
#> 154 0.29 12.78 0.00 10
#> 155 0.30 12.39 0.00 7
#> 156 0.36 11.18 0.00 2
#> 157 0.27 11.05 40.32 6
#> 158 0.23 10.66 183.83 1
#> 159 0.23 10.39 45.96 3
#> 160 0.22 10.30 46.39 3
#> 161 0.21 9.88 47.04 11
#> 162 0.22 9.70 43.68 11
#> 163 0.21 9.58 69.51 10
#> 164 0.20 9.28 185.57 3
#> 165 0.27 9.00 99.23 15
#> 166 0.21 8.69 38.61 3
#> 167 0.19 8.45 227.07 3
#> 168 0.18 8.18 229.79 8
#> 169 0.17 8.13 48.39 16
#> 170 0.21 8.08 99.09 16
#> 171 0.18 8.05 45.24 3
#> 172 0.17 8.05 88.81 9
#> 173 0.20 7.98 40.32 3
#> 174 0.17 7.72 137.87 2
#> 175 0.17 7.72 505.53 5
#> 176 0.17 7.54 0.00 4
#> 177 0.18 7.38 364.80 8
#> 178 0.16 7.17 229.79 6
#> 179 0.17 7.16 341.10 8
#> 180 0.16 7.04 44.66 11
#> 181 0.14 6.73 92.79 11
#> 182 0.15 6.36 178.29 7
#> 183 0.16 6.29 201.60 1
#> 184 0.14 6.25 183.83 1
#> 185 0.15 6.19 40.75 4
#> 186 0.15 6.15 106.36 5
#> 187 0.15 6.12 41.89 1
#> 188 0.16 6.10 116.07 3
#> 189 0.14 5.88 224.81 8
#> 190 0.13 5.88 44.51 4
#> 191 0.13 5.73 0.00 10
#> 192 0.13 5.70 181.11 1
#> 193 0.14 5.51 118.08 3
#> 194 0.14 5.48 40.32 1
#> 195 0.14 5.16 93.04 8
#> 196 0.15 4.72 31.05 1
#> 197 0.10 4.71 90.49 10
#> 198 0.11 4.61 12.88 7
#> 199 0.10 4.19 64.99 16
#> 200 0.09 4.18 46.39 16
#> 201 0.08 4.06 48.39 3
#> 202 0.09 3.94 92.79 1
#> 203 0.09 3.87 42.97 1
#> 204 0.10 3.86 119.53 5
#> 205 0.08 3.83 45.02 9
#> 206 0.09 3.73 51.53 11
#> 207 0.08 3.58 186.53 5
#> 208 0.09 3.51 113.09 8
#> 209 0.08 3.45 86.17 3
#> 210 0.07 3.34 46.39 11
#> 211 0.07 3.29 47.04 10
#> 212 0.07 3.24 90.96 8
#> 213 0.08 3.22 124.96 4
#> 214 0.07 3.12 0.00 5
#> 215 0.07 3.09 46.87 3
#> 216 0.06 2.93 52.31 11
#> 217 0.06 2.90 48.39 2
#> 218 0.07 2.88 87.35 7
#> 219 0.06 2.72 46.87 6
#> 220 0.06 2.70 43.62 2
#> 221 0.06 2.67 45.96 11
#> 222 0.06 2.67 91.92 4
#> 223 0.06 2.63 45.41 3
#> 224 0.07 2.56 23.44 11
#> 225 0.06 2.55 354.05 13
#> 226 0.06 2.45 44.51 5
#> 227 0.06 2.43 0.00 11
#> 228 0.06 2.42 59.49 7
#> 229 0.06 2.41 43.09 11
#> 230 0.06 2.41 62.24 4
#> 231 0.05 2.27 43.68 7
#> 232 0.05 2.23 44.51 4
#> 233 0.05 2.18 40.32 2
#> 234 0.05 2.14 44.51 3
#> 235 0.04 1.96 90.96 10
#> 236 0.04 1.93 0.00 7
#> 237 0.04 1.75 91.92 8
#> 238 0.04 1.71 112.19 7
#> 239 0.04 1.69 39.97 5
#> 240 0.04 1.69 46.87 5
#> 241 0.04 1.66 115.62 10
#> 242 0.04 1.65 39.36 2
#> 243 0.03 1.65 48.39 4
#> 244 0.04 1.57 88.61 5
#> 245 0.04 1.56 404.05 13
#> 246 0.03 1.56 45.96 1
#> 247 0.04 1.51 41.89 4
#> 248 0.04 1.47 40.75 5
#> 249 0.03 1.42 57.76 11
#> 250 0.03 1.41 41.59 10
#> 251 0.03 1.34 44.51 6
#> 252 0.03 1.32 69.37 5
#> 253 0.03 1.24 44.19 8
#> 254 0.03 1.23 44.09 5
#> 255 0.03 1.20 46.45 5
#> 256 0.03 1.17 90.01 3
#> 257 0.03 1.14 45.62 3
#> 258 0.03 1.13 43.62 3
#> 259 0.02 1.11 46.39 3
#> 260 0.02 1.04 0.00 5
#> 261 0.02 0.94 46.87 4
#> 262 0.02 0.89 80.64 5
#> 263 0.02 0.87 145.17 3
#> 264 0.02 0.85 44.36 9
#> 265 0.02 0.81 0.00 3
#> 266 0.02 0.74 45.96 1
#> 267 0.02 0.73 40.32 1
#> 268 0.01 0.69 133.87 13
#> 269 0.01 0.67 44.51 8
#> 270 0.01 0.67 46.18 7
#> 271 0.02 0.66 59.88 8
#> 272 0.01 0.64 0.00 5
#> 273 0.01 0.63 45.24 1
#> 274 0.01 0.57 62.94 7
#> 275 0.01 0.54 0.00 23
#> 276 0.01 0.53 44.09 8
#> 277 0.01 0.48 48.39 2
#> 278 0.01 0.47 39.36 1
#> 279 0.01 0.47 46.87 1
#> 280 0.01 0.46 91.92 2
#> 281 0.01 0.45 44.51 1
#> 282 0.01 0.45 44.51 5
#> 283 0.01 0.45 89.02 1
#> 284 0.01 0.45 133.54 13
#> 285 0.01 0.43 42.64 10
#> 286 0.01 0.42 41.89 5
#> 287 0.01 0.39 138.31 13
#> 288 0.01 0.35 44.01 8
#> 289 0.01 0.34 41.89 1
#> 290 0.01 0.33 55.22 1
#> 291 0.01 0.33 40.75 2
#> 292 0.01 0.32 40.32 1
#> 293 0.01 0.28 91.92 1
#> 294 0.01 0.24 40.32 2
#> 295 0.00 0.22 44.51 1
#> 296 0.00 0.22 43.68 1
#> 297 0.00 0.18 44.01 1
#> 298 0.00 0.17 41.85 1
#> 299 0.00 0.10 0.00 2
#> 300 0.00 0.09 45.96 2
#> 301 0.00 0.08 0.00 5
#> 302 0.00 0.08 120.96 1
#> 303 0.00 0.08 39.36 1
#>
#> $haul
#> year station haul stratum vessel_name date_time
#> 1 2002 320-73 27 721 VESTERAALEN 2002-05-23 14:57:45
#> 2 2002 320-74 28 721 VESTERAALEN 2002-05-23 15:53:07
#> 3 2002 321-74 23 721 VESTERAALEN 2002-05-22 12:37:05
#> 4 2002 324-73 16 721 VESTERAALEN 2002-05-20 08:47:20
#> 5 2004 320-73 6 721 GLADIATOR 2004-06-08 07:16:35
#> 6 2004 320-74 7 721 SEA STORM 2004-06-08 12:14:10
#> 7 2004 321-74 1 721 GLADIATOR 2004-06-06 14:06:11
#> 8 2004 324-73 5 721 GLADIATOR 2004-06-07 17:09:12
#> 9 2004 326-76 4 721 SEA STORM 2004-06-07 17:51:19
#> 10 2006 320-74 7 722 GLADIATOR 2006-06-07 14:18:43
#> 11 2006 321-74 7 721 SEA STORM 2006-06-08 09:39:09
#> 12 2006 324-73 6 721 SEA STORM 2006-06-08 07:12:11
#> 13 2006 325-76 5 721 SEA STORM 2006-06-07 16:25:03
#> 14 2010 320-73 5 721 OCEAN EXPLORER 2010-06-10 13:05:42
#> 15 2010 324-73 3 721 OCEAN EXPLORER 2010-06-10 07:12:59
#> 16 2010 325-76 7 721 SEA STORM 2010-06-11 10:47:39
#> 17 2010 326-76 3 722 SEA STORM 2010-06-10 11:11:17
#> 18 2010 326-76 4 721 SEA STORM 2010-06-10 14:47:59
#> 19 2012 320-73 7 721 OCEAN EXPLORER 2012-06-10 07:03:03
#> 20 2012 320-74 1 721 SEA STORM 2012-06-08 07:27:27
#> 21 2012 320-74 7 722 SEA STORM 2012-06-09 08:11:36
#> 22 2012 321-74 6 721 OCEAN EXPLORER 2012-06-09 16:34:38
#> 23 2012 326-76 4 721 SEA STORM 2012-06-08 14:16:40
#> 24 2012 326-76 1 722 OCEAN EXPLORER 2012-06-09 07:10:55
#> 25 2014 320-74 3 722 SEA STORM 2014-06-11 16:00:45
#> 26 2014 320-74 6 721 SEA STORM 2014-06-12 13:26:56
#> 27 2014 321-74 1 721 ALASKA PROVIDER 2014-06-11 17:40:03
#> 28 2014 326-76 5 722 ALASKA PROVIDER 2014-06-12 14:34:14
#> 29 2016 320-73 3 721 ALASKA PROVIDER 2016-06-07 15:15:11
#> 30 2016 320-74 5 721 SEA STORM 2016-06-07 18:04:38
#> 31 2016 320-74 7 722 SEA STORM 2016-06-08 09:23:26
#> 32 2016 324-73 4 721 SEA STORM 2016-06-07 16:05:45
#> 33 2018 320-73 10 721 SEA STORM 2018-06-11 17:28:48
#> 34 2018 320-74 9 722 SEA STORM 2018-06-11 15:08:22
#> 35 2018 324-73 1 721 OCEAN EXPLORER 2018-06-09 07:27:06
#> 36 2018 325-76 2 721 OCEAN EXPLORER 2018-06-09 11:58:02
#> 37 2022 324-73 6 721 OCEAN EXPLORER 2022-06-11 08:10:18
#> 38 2022 326-76 5 722 OCEAN EXPLORER 2022-06-10 15:59:58
#> 39 2024 321-74 6 721 OCEAN EXPLORER 2024-06-08 15:29:17
#> latitude_dd_start longitude_dd_start bottom_temperature_c
#> 1 54.21785 -166.0437 4.3
#> 2 54.23024 -166.0035 4.5
#> 3 54.24220 -165.9348 4.7
#> 4 54.20549 -165.7466 4.5
#> 5 54.20921 -166.0613 5.2
#> 6 54.23151 -165.9993 5.2
#> 7 54.25199 -165.9398 5.0
#> 8 54.20250 -165.7467 5.2
#> 9 54.34954 -165.6114 4.4
#> 10 54.25761 -165.9996 4.1
#> 11 54.25770 -165.9099 5.1
#> 12 54.21174 -165.7529 4.9
#> 13 54.34783 -165.6215 4.6
#> 14 54.21217 -166.0394 4.7
#> 15 54.19405 -165.7223 4.7
#> 16 54.34708 -165.6544 4.1
#> 17 54.34305 -165.5495 4.2
#> 18 54.33688 -165.5711 4.2
#> 19 54.21403 -166.0355 4.1
#> 20 54.24227 -165.9876 4.0
#> 21 54.24643 -166.0219 3.7
#> 22 54.24130 -165.9455 4.1
#> 23 54.33457 -165.5654 3.7
#> 24 54.34417 -165.5489 3.7
#> 25 54.24728 -166.0211 4.5
#> 26 54.21945 -166.0375 5.0
#> 27 54.23909 -165.9084 5.7
#> 28 54.34741 -165.5540 4.4
#> 29 54.20566 -166.0491 6.3
#> 30 54.22642 -166.0167 6.4
#> 31 54.25784 -166.0004 6.2
#> 32 54.21666 -165.7556 6.4
#> 33 54.21682 -166.0323 5.6
#> 34 54.24662 -166.0222 4.5
#> 35 54.21011 -165.7440 5.3
#> 36 54.35293 -165.5989 5.0
#> 37 54.21109 -165.7537 5.3
#> 38 54.34763 -165.5462 4.7
#> 39 54.23554 -165.8981 4.7
#> surface_temperature_c depth_m distance_fished_km net_width_m net_height_m
#> 1 4.8 82 1.442 13.258 8.361
#> 2 5.0 71 1.438 14.918 7.530
#> 3 4.8 62 1.454 15.190 7.503
#> 4 5.2 93 1.425 16.331 7.773
#> 5 5.8 82 1.491 15.010 7.998
#> 6 5.6 71 1.448 15.475 7.723
#> 7 5.4 89 1.807 15.348 NA
#> 8 6.6 91 1.578 16.398 7.396
#> 9 6.1 83 1.257 14.408 8.502
#> 10 5.5 109 1.454 15.565 6.925
#> 11 5.4 90 1.425 14.972 7.218
#> 12 6.4 92 1.473 16.206 5.371
#> 13 6.1 85 1.408 15.454 6.992
#> 14 4.9 61 1.487 15.280 7.434
#> 15 5.2 92 1.530 15.329 7.384
#> 16 4.9 86 1.364 17.091 6.945
#> 17 5.2 108 1.463 15.670 6.639
#> 18 5.2 89 1.441 15.889 6.640
#> 19 4.2 60 1.615 14.175 7.906
#> 20 4.6 95 1.498 14.431 7.739
#> 21 4.3 109 1.426 15.822 6.996
#> 22 4.3 62 1.573 14.420 7.494
#> 23 4.2 96 1.436 14.805 7.375
#> 24 4.0 112 1.575 14.264 7.755
#> 25 6.4 110 1.543 16.074 6.041
#> 26 6.4 73 1.501 14.725 6.267
#> 27 6.3 69 1.578 15.142 7.007
#> 28 6.3 122 1.277 16.183 6.198
#> 29 7.1 61 1.526 15.209 5.907
#> 30 7.4 74 1.515 14.666 7.647
#> 31 6.9 110 1.441 15.281 7.178
#> 32 7.6 93 1.436 17.091 6.704
#> 33 5.8 63 1.436 15.560 6.568
#> 34 7.0 109 1.451 17.671 6.146
#> 35 NA 96 1.549 16.402 4.755
#> 36 6.2 83 1.321 16.317 5.575
#> 37 6.2 91 1.438 19.682 5.069
#> 38 6.0 129 1.508 16.585 5.643
#> 39 4.8 58 1.737 18.539 7.608
#> area_swept_km2 duration_hr total_weight_kg
#> 1 0.01911804 0.260 77.91
#> 2 0.02145208 0.260 217.12
#> 3 0.02208626 0.260 4055.00
#> 4 0.02327167 0.290 183.04
#> 5 0.02237991 0.270 270.23
#> 6 0.02240780 0.270 538.84
#> 7 0.02773384 0.350 339.77
#> 8 0.02587604 0.280 988.46
#> 9 0.01811086 0.230 11793.00
#> 10 0.02263151 0.277 541.80
#> 11 0.02133510 0.264 268.14
#> 12 0.02387144 0.270 401.73
#> 13 0.02175923 0.262 577.60
#> 14 0.02272136 0.269 407.52
#> 15 0.02345337 0.286 434.93
#> 16 0.02331212 0.248 3038.58
#> 17 0.02292521 0.262 3475.82
#> 18 0.02289605 0.261 3475.82
#> 19 0.02289262 0.289 101.15
#> 20 0.02161764 0.274 89.89
#> 21 0.02256217 0.267 89.89
#> 22 0.02268266 0.286 496.86
#> 23 0.02125998 0.269 189.13
#> 24 0.02246580 0.282 189.13
#> 25 0.02480218 0.279 872.01
#> 26 0.02210222 0.278 872.01
#> 27 0.02389408 0.289 252.24
#> 28 0.02066569 0.250 131.01
#> 29 0.02320893 0.272 590.05
#> 30 0.02221899 0.274 410.23
#> 31 0.02201992 0.265 410.23
#> 32 0.02454268 0.269 111.26
#> 33 0.02234416 0.294 32.12
#> 34 0.02564062 0.269 867.17
#> 35 0.02540670 0.274 571.17
#> 36 0.02155476 0.239 1735.27
#> 37 0.02830272 0.258 1017.75
#> 38 0.02501018 0.274 941.97
#> 39 0.03220224 0.304 308.79
#>
## for default 10 years and nearby stations for Bering Flounder (0 results returned!) ---
get_catch_haul_history(
survey = "AI",
species_codes = 10140, # Bering flounder which would be VERY unlikely to be found
years = NA, # default
station = "324-73",
grid_buffer = 3)
#> [1] "Your quiery returned 0 results."